python给数据集多个子文件夹中的彩色图像加噪
时间: 2023-07-30 17:09:36 浏览: 43
你可以使用Python的PIL库来给数据集中的彩色图像加噪。具体步骤如下:
1. 导入PIL库和其他必要的库:
```python
from PIL import Image, ImageFilter
import os
import random
```
2. 定义一个函数来给图像加噪。这里我们使用高斯滤波器来模拟噪声。函数接收一个图像对象和噪声因子作为参数,并返回一个加了噪声的图像对象:
```python
def add_noise(image, factor):
# 计算噪声的标准差
noise = int(factor * random.randint(1, 10))
# 应用高斯滤波器模拟噪声
image = image.filter(ImageFilter.GaussianBlur(radius=noise))
return image
```
3. 遍历数据集中的每个子文件夹,读取其中的彩色图像并加噪:
```python
# 数据集根目录
root_dir = 'dataset'
# 噪声因子
noise_factor = 0.05
# 遍历每个子文件夹
for subdir in os.listdir(root_dir):
subdir_path = os.path.join(root_dir, subdir)
# 如果子文件夹不是文件夹则跳过
if not os.path.isdir(subdir_path):
continue
# 遍历子文件夹中的每个图像文件
for filename in os.listdir(subdir_path):
file_path = os.path.join(subdir_path, filename)
# 如果文件不是图像文件则跳过
if not file_path.endswith('.jpg') and not file_path.endswith('.png'):
continue
# 打开图像文件
image = Image.open(file_path)
# 给图像加噪
noisy_image = add_noise(image, noise_factor)
# 保存加了噪的图像
noisy_image.save(file_path)
```
这样,就可以给数据集中的所有彩色图像加上噪声了。注意,这里只给了一个简单的示例函数来模拟噪声,你可以根据需要使用其他的噪声生成方法。同时,为了防止重复添加噪声,你可以在加噪函数中添加一些随机性,比如每次使用不同的噪声半径。
相关推荐
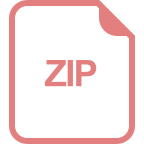














