vue实现图片标注功能
时间: 2023-08-17 18:09:41 浏览: 82
Vue可以利用其动态数据绑定和组件化的特性来实现图片标注功能。下面是一个简单的示例代码:
1. 首先,创建一个Vue组件,用于展示图片和标注数据:
```vue
<template>
<div>
<img :src="imageUrl" @click="handleImageClick" />
<div v-for="annotation in annotations" :key="annotation.id" :style="annotation.style"></div>
</div>
</template>
<script>
export default {
data() {
return {
imageUrl: 'path_to_your_image',
annotations: []
};
},
methods: {
handleImageClick(event) {
const rect = event.target.getBoundingClientRect();
const x = event.clientX - rect.left;
const y = event.clientY - rect.top;
// 创建新的标注对象,可以根据需要添加其他属性
const newAnnotation = {
id: this.annotations.length + 1,
style: {
position: 'absolute',
left: x + 'px',
top: y + 'px',
width: '10px',
height: '10px',
background: 'red'
}
};
// 更新标注数据
this.annotations.push(newAnnotation);
}
}
};
</script>
```
2. 在父组件中使用该图片标注组件:
```vue
<template>
<div>
<image-annotation></image-annotation>
</div>
</template>
<script>
import ImageAnnotation from './ImageAnnotation.vue';
export default {
components: {
ImageAnnotation
}
};
</script>
```
在上述代码中,点击图片时会根据点击位置创建新的标注对象,并将其添加到`annotations`数组中。每个标注对象都有一个唯一的id和对应的样式,用于在页面上显示标注。
你可以根据实际需求修改标注的样式和数据结构。此外,你还可以添加其他功能,比如删除标注、保存标注数据等。希望这个示例对你有所帮助!
相关推荐
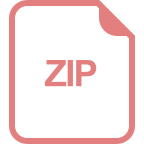
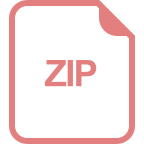
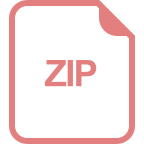
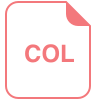
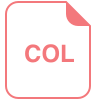
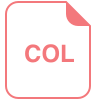
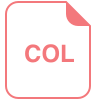
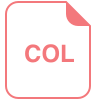









