用c语言写出一个打飞机小游戏代码
时间: 2024-01-30 09:01:47 浏览: 19
以下是一个简单的打飞机小游戏的代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
#include <windows.h>
#define SCREEN_WIDTH 80
#define SCREEN_HEIGHT 25
// 定义飞机的位置和状态
int planeX = SCREEN_WIDTH / 2;
int planeY = SCREEN_HEIGHT - 2;
int planeState = 0; // 0: 未被击中,1: 已被击中
// 定义子弹的位置和状态
int bulletX = -1;
int bulletY = -1;
int bulletState = 0; // 0: 不存在,1: 存在
// 定义敌机的位置和状态
int enemyX = -1;
int enemyY = -1;
int enemyState = 0; // 0: 不存在,1: 存在
// 清屏函数
void clearScreen() {
system("cls");
}
// 设置光标位置函数
void setCursorPosition(int x, int y) {
COORD position = {x, y};
SetConsoleCursorPosition(GetStdHandle(STD_OUTPUT_HANDLE), position);
}
// 显示飞机函数
void showPlane() {
setCursorPosition(planeX, planeY);
printf("^");
}
// 移动飞机函数
void movePlane(int x, int y) {
if (planeState == 1) {
return;
}
if (x < 0 || x >= SCREEN_WIDTH || y < 0 || y >= SCREEN_HEIGHT) {
return;
}
planeX = x;
planeY = y;
}
// 显示子弹函数
void showBullet() {
if (bulletState == 0) {
return;
}
setCursorPosition(bulletX, bulletY);
printf("|");
}
// 移动子弹函数
void moveBullet() {
if (bulletState == 0) {
return;
}
bulletY--;
if (bulletY < 0) {
bulletState = 0;
}
}
// 显示敌机函数
void showEnemy() {
if (enemyState == 0) {
return;
}
setCursorPosition(enemyX, enemyY);
printf("V");
}
// 移动敌机函数
void moveEnemy() {
if (enemyState == 0) {
return;
}
enemyY++;
if (enemyY >= SCREEN_HEIGHT) {
enemyState = 0;
}
}
// 发射子弹函数
void fireBullet() {
if (bulletState == 1) {
return;
}
bulletX = planeX;
bulletY = planeY - 1;
bulletState = 1;
}
// 发射敌机函数
void fireEnemy() {
if (enemyState == 1) {
return;
}
enemyX = rand() % SCREEN_WIDTH;
enemyY = 0;
enemyState = 1;
}
// 判断是否碰撞函数
int isCollide(int x1, int y1, int x2, int y2) {
if (x1 == x2 && y1 == y2) {
return 1;
}
return 0;
}
// 游戏循环函数
void gameLoop() {
while (1) {
clearScreen();
showPlane();
showBullet();
showEnemy();
if (_kbhit()) {
int ch = _getch();
switch (ch) {
case 'a':
movePlane(planeX - 1, planeY);
break;
case 'd':
movePlane(planeX + 1, planeY);
break;
case 's':
movePlane(planeX, planeY + 1);
break;
case ' ':
fireBullet();
break;
}
}
moveBullet();
moveEnemy();
if (isCollide(bulletX, bulletY, enemyX, enemyY)) {
bulletState = 0;
enemyState = 0;
}
if (isCollide(planeX, planeY, enemyX, enemyY)) {
planeState = 1;
break;
}
Sleep(100);
}
clearScreen();
setCursorPosition(SCREEN_WIDTH / 2 - 5, SCREEN_HEIGHT / 2);
printf("Game Over");
}
// 主函数
int main() {
gameLoop();
return 0;
}
```
这个小游戏使用了 Windows 平台的控制台窗口作为游戏界面,使用了 conio.h 库来获取键盘输入,使用了 windows.h 库来设置控制台窗口的光标位置和清屏。游戏循环中使用了 Sleep() 函数来控制游戏速度。
相关推荐
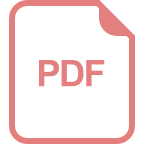














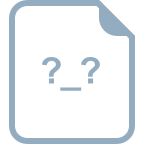