在vue项目中如何利用canvas实现立体字效果
时间: 2024-01-24 08:17:08 浏览: 36
要在 Vue 项目中实现立体字效果,可以通过使用 `canvas` 绘制文字并添加阴影来实现。下面是一个简单的实现步骤:
1. 在 Vue 组件中添加一个 `canvas` 元素,并为其设置一个 `ref` 属性:
```html
<template>
<div>
<canvas ref="canvas"></canvas>
</div>
</template>
```
2. 在组件的 `mounted` 钩子函数中获取 `canvas` 元素和绘图上下文,并设置字体样式:
```js
mounted() {
const canvas = this.$refs.canvas;
const ctx = canvas.getContext('2d');
ctx.font = 'bold 48px Arial';
}
```
3. 绘制文字和阴影:
```js
// 绘制立体字
ctx.fillStyle = '#333';
ctx.fillText('Hello', 50, 50);
ctx.fillStyle = '#666';
ctx.fillText('Hello', 52, 52);
// 添加阴影
ctx.shadowColor = 'rgba(0, 0, 0, 0.5)';
ctx.shadowOffsetX = 5;
ctx.shadowOffsetY = 5;
ctx.shadowBlur = 5;
```
完整代码如下:
```html
<template>
<div>
<canvas ref="canvas"></canvas>
</div>
</template>
<script>
export default {
mounted() {
const canvas = this.$refs.canvas;
const ctx = canvas.getContext('2d');
ctx.font = 'bold 48px Arial';
// 绘制立体字
ctx.fillStyle = '#333';
ctx.fillText('Hello', 50, 50);
ctx.fillStyle = '#666';
ctx.fillText('Hello', 52, 52);
// 添加阴影
ctx.shadowColor = 'rgba(0, 0, 0, 0.5)';
ctx.shadowOffsetX = 5;
ctx.shadowOffsetY = 5;
ctx.shadowBlur = 5;
},
};
</script>
```
相关推荐
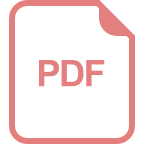
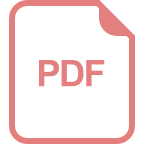
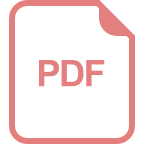














