点云如何与RGB图像融合,并输出融合后的文件,给出代码示例
时间: 2024-05-13 07:20:34 浏览: 126
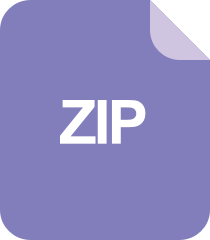
从单个RGB图像构建3D点云模型的Pytorch代码_Python_Shell_下载.zip
点云与RGB图像的融合可以通过将RGB图像投影到点云上来实现。具体的步骤如下:
1. 读取点云数据和RGB图像数据;
2. 将RGB图像转换为与点云相同的坐标系;
3. 将RGB图像投影到点云上,得到每个点的颜色信息;
4. 将点云和颜色信息合并为一个点云数据,并输出文件。
以下是一个简单的代码示例,使用PCL库实现点云和RGB图像的融合:
```cpp
#include <iostream>
#include <pcl/io/pcd_io.h>
#include <pcl/point_types.h>
#include <pcl/visualization/pcl_visualizer.h>
#include <pcl/visualization/cloud_viewer.h>
#include <pcl/filters/voxel_grid.h>
#include <pcl/features/normal_3d.h>
#include <pcl/kdtree/kdtree.h>
#include <pcl/surface/mls.h>
#include <pcl/filters/passthrough.h>
#include <pcl/filters/statistical_outlier_removal.h>
#include <opencv2/opencv.hpp>
using namespace std;
using namespace cv;
typedef pcl::PointXYZRGBA PointT;
typedef pcl::PointCloud<PointT> PointCloud;
int main(int argc, char** argv)
{
// 读取点云和RGB图像
PointCloud::Ptr cloud(new PointCloud);
pcl::io::loadPCDFile<PointT>("input_cloud.pcd", *cloud);
Mat rgb_image = imread("rgb_image.png");
// 将RGB图像转换为与点云相同的坐标系
float fx = 525.0;
float fy = 525.0;
float cx = 319.5;
float cy = 239.5;
for (int i = 0; i < cloud->size(); ++i)
{
PointT& p = cloud->points[i];
int x = static_cast<int>(p.x * fx / p.z + cx);
int y = static_cast<int>(p.y * fy / p.z + cy);
if (x >= 0 && x < rgb_image.cols && y >= 0 && y < rgb_image.rows)
{
p.r = rgb_image.at<Vec3b>(y, x)[2];
p.g = rgb_image.at<Vec3b>(y, x)[1];
p.b = rgb_image.at<Vec3b>(y, x)[0];
}
}
// 输出融合后的点云
pcl::io::savePCDFileBinary("output_cloud.pcd", *cloud);
return 0;
}
```
上述代码中,我们假设点云和RGB图像都是在相机坐标系下获取的,因此可以通过相机内参将RGB图像转换为点云坐标系下的图像。在代码中,我们使用了OpenCV库读取和处理RGB图像,并使用PCL库读取和处理点云数据。最后,将融合后的点云数据输出到文件中。
阅读全文
相关推荐
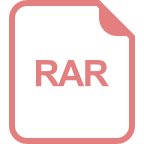



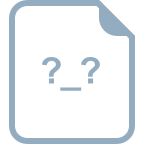
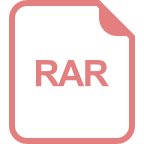
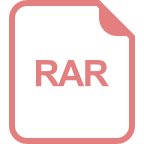
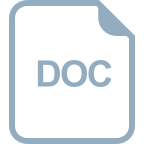
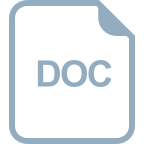
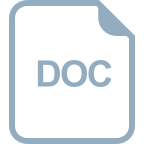
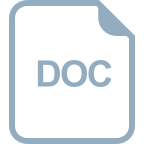
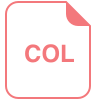
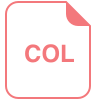
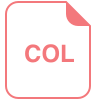
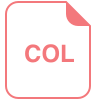
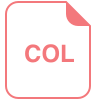