python, 我想把索引去掉,把其中tuple的两个数值拆开作为分别的两列
时间: 2024-03-01 20:51:00 浏览: 149
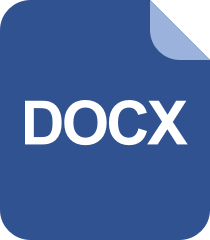
python初学list与tuple教程
可以使用 Pandas 中的 `apply()` 函数和 `pd.Series()` 函数来实现。
假设你的 DataFrame 名称为 `df`,其中包含一个名为 `tuple_col` 的列,该列的每个元素都是一个长度为2的 tuple。现在你想要将 `tuple_col` 列中的 tuple 拆分为两列,并且去掉 DataFrame 的索引。你可以按照以下步骤进行操作:
1. 将 `tuple_col` 列中的 tuple 拆分为两列:
```python
df[['col1', 'col2']] = df['tuple_col'].apply(pd.Series)
```
2. 去掉 DataFrame 的索引:
```python
df.reset_index(drop=True, inplace=True)
```
在上述代码中,`apply()` 函数将 `tuple_col` 列中的每个元素(即 tuple)作为输入,并将其转换为一个 Pandas Series,其中包含两个元素。`pd.Series()` 函数将 Series 转换为 DataFrame 中的两列。
`reset_index()` 函数将 DataFrame 的索引重置为默认的数字索引,并将 `drop` 参数设置为 `True`,以便去掉原有索引。`inplace` 参数的值设置为 `True`,以便在原有 DataFrame 上直接修改,而不是返回一个新的 DataFrame。
阅读全文
相关推荐
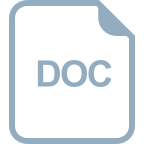
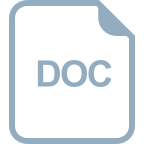


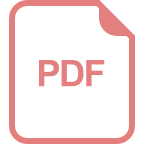
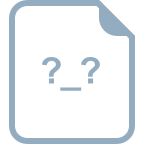
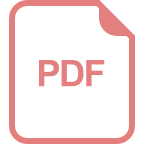
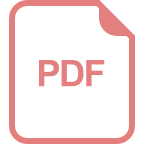
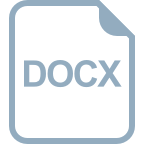
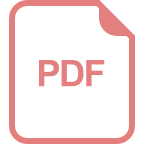
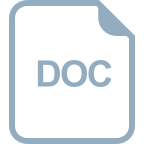





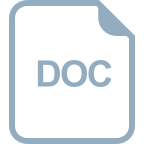