python轮廓检测教程
时间: 2023-10-03 07:08:53 浏览: 103
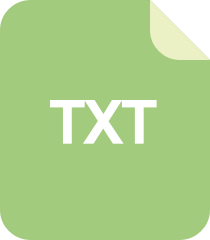
轮廓检测代码
轮廓检测是一种常用的图像处理技术,可以用于图像分割、目标识别、形状分析等领域。在Python中,OpenCV库提供了轮廓检测的函数。
以下是Python轮廓检测的教程:
1. 导入OpenCV库和numpy库
```python
import cv2
import numpy as np
```
2. 读取图像并将其转换为灰度图像
```python
img = cv2.imread('image.jpg')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
```
3. 对灰度图像进行二值化处理
```python
ret, thresh = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY)
```
其中,第一个参数是原始灰度图像,第二个参数是阈值,第三个参数是最大值,第四个参数是二值化类型。
4. 进行轮廓检测
```python
contours, hierarchy = cv2.findContours(thresh, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
```
其中,第一个参数是二值化后的图像,第二个参数是轮廓检测模式,第三个参数是轮廓近似方法。
5. 绘制轮廓
```python
cv2.drawContours(img, contours, -1, (0, 0, 255), 2)
```
其中,第一个参数是原始图像,第二个参数是轮廓列表,第三个参数是轮廓索引(-1表示绘制所有轮廓),第四个参数是颜色,第五个参数是线条宽度。
6. 显示图像
```python
cv2.imshow('image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
完整代码如下:
```python
import cv2
import numpy as np
img = cv2.imread('image.jpg')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
ret, thresh = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY)
contours, hierarchy = cv2.findContours(thresh, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
cv2.drawContours(img, contours, -1, (0, 0, 255), 2)
cv2.imshow('image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
运行结果如下图所示:

阅读全文
相关推荐
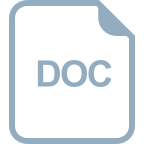
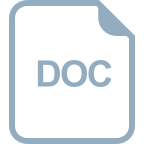
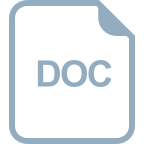
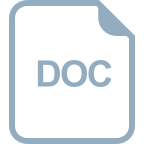
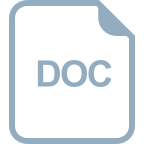
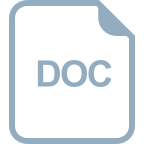
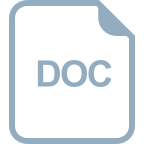
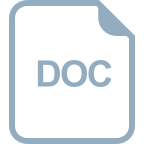
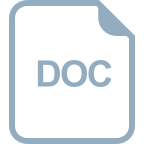
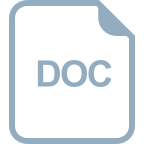
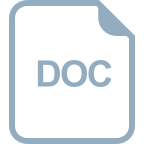
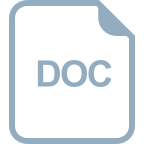
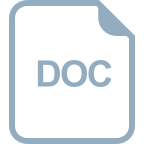
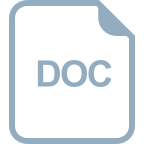
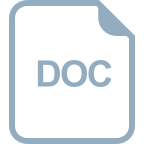
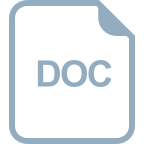