写一个抓取个税信息的爬虫
时间: 2023-06-27 22:01:08 浏览: 135
抓取个税信息需要登录个人所得税官网并输入相关信息,因此需要使用模拟登录的方式进行爬取。以下是一个基于Python的抓取个税信息的爬虫示例:
```python
import requests
from bs4 import BeautifulSoup
# 登录所需信息
username = 'your_username'
password = 'your_password'
# 其他所需信息
year = '2021'
month = '7'
# 登录页面和个人所得税信息页面的URL
login_url = 'https://www.chinatax.gov.cn/sso/login'
info_url = 'https://www.chinatax.gov.cn/sso/anonymous/queryTaxRecordDetail'
# 创建会话对象
session = requests.Session()
# 获取登录页面的HTML代码
response = session.get(login_url)
html = response.text
# 使用BeautifulSoup解析HTML代码,获取登录所需的参数
soup = BeautifulSoup(html, 'html.parser')
lt = soup.find(attrs={'name': 'lt'})['value']
execution = soup.find(attrs={'name': 'execution'})['value']
# 构造登录所需的数据
data = {
'username': username,
'password': password,
'captcha': '',
'lt': lt,
'execution': execution,
'_eventId': 'submit'
}
# 发送登录请求
response = session.post(login_url, data=data)
# 获取个人所得税信息
params = {
'nsrmc': '', # 纳税人名称,可为空
'nsrsbh': '', # 纳税人识别号,可为空
'skssqq': year + '01', # 税款所属期起
'skssqz': year + month, # 税款所属期止
'nsrdzdah': '', # 纳税人电子档案号,可为空
'sblx': '02', # 申报类型,固定为02(个人所得税)
'yzm': '', # 验证码,可为空
}
headers = {
'Referer': 'https://www.chinatax.gov.cn/sso/anonymous/showPage?params=%7B%22service%22%3A%22https%3A%2F%2Fetax.gdltax.gov.cn%2F%22%2C%22path%22%3A%22%2Fsqd%2Findex.html%22%7D',
'X-Requested-With': 'XMLHttpRequest',
'Content-Type': 'application/x-www-form-urlencoded; charset=UTF-8'
}
response = session.post(info_url, params=params, headers=headers)
# 输出个人所得税信息
print(response.text)
```
需要注意的是,此爬虫仅供学习和研究使用,不得用于任何商业用途。
阅读全文
相关推荐



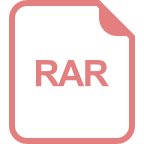



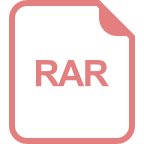




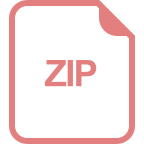


