pcm转mp4
时间: 2023-09-10 21:07:39 浏览: 184
PCM是一种无损音频编码格式,而MP4是一种常用的视频编码格式,它支持多种音频编码格式,包括AAC、MP3、AC3等。如果你想将PCM格式的音频转换为MP4格式的音频,可以按照以下步骤进行:
1. 将PCM格式的音频文件转换为WAV格式。由于PCM格式的音频文件没有文件头信息,无法直接被播放器或编码器识别,因此需要将其转换为WAV格式。可以使用FFmpeg等工具进行转换,命令如下:
```
ffmpeg -f s16le -ar 44100 -ac 2 -i input.pcm output.wav
```
其中,`-f`参数表示输入的音频格式为s16le(即PCM),`-ar`参数表示采样率为44100Hz,`-ac`参数表示声道数为2(双声道),`-i`参数后面为输入文件名,`output.wav`为输出文件名。
2. 将WAV文件转换为MP4格式。可以使用FFmpeg等工具进行转换,命令如下:
```
ffmpeg -i input.wav -c:a aac -b:a 128k output.mp4
```
其中,`-i`参数后面为输入文件名,`-c:a`参数表示使用AAC编码格式,`-b:a`参数表示音频比特率为128k,`output.mp4`为输出文件名。
需要注意的是,由于MP4是一种视频编码格式,因此在进行转换时需要同时指定视频编码格式和音频编码格式。如果输入文件只包含音频数据,则需要在转换时指定一个虚拟的视频流。
希望这个回答能够帮助到你。
相关问题
安卓 pcm 转 mp3 mediacodec
要使用 MediaCodec 进行安卓 PCM 转 MP3,你需要进行以下步骤:
1. 创建一个 MediaCodec 实例并配置它:
```
MediaCodec codec = MediaCodec.createEncoderByType("audio/mp4a-latm");
MediaFormat format = MediaFormat.createAudioFormat("audio/mp4a-latm", sampleRate, channelCount);
format.setInteger(MediaFormat.KEY_BIT_RATE, bitRate);
format.setInteger(MediaFormat.KEY_AAC_PROFILE, MediaCodecInfo.CodecProfileLevel.AACObjectLC);
codec.configure(format, null, null, MediaCodec.CONFIGURE_FLAG_ENCODE);
```
这个代码片段中,我们创建了一个编码器的实例,并配置了一些参数,比如采样率、声道数、比特率和编码器配置参数等。
2. 创建一个输出流:
```
String outputPath = Environment.getExternalStorageDirectory() + "/output.mp3";
FileOutputStream fos = new FileOutputStream(outputPath);
```
这个代码片段中,我们创建了一个文件输出流,用于将编码后的数据写入到文件中。
3. 启动编码器:
```
codec.start();
```
4. 将 PCM 数据传递给编码器:
```
ByteBuffer[] codecInputBuffers = codec.getInputBuffers();
ByteBuffer[] codecOutputBuffers = codec.getOutputBuffers();
int inputBufferIndex = codec.dequeueInputBuffer(-1);
if (inputBufferIndex >= 0) {
ByteBuffer inputBuffer = codecInputBuffers[inputBufferIndex];
inputBuffer.clear();
inputBuffer.put(input);
codec.queueInputBuffer(inputBufferIndex, 0, input.length, 0, 0);
}
```
这个代码片段中,我们获取编码器的输入和输出缓冲区,并从输入缓冲区中获取一个可用的缓冲区(如果没有可用的,就会一直等待),将 PCM 数据写入该缓冲区,然后将缓冲区提交给编码器进行编码。
5. 从编码器获取编码后的数据:
```
MediaCodec.BufferInfo bufferInfo = new MediaCodec.BufferInfo();
int outputBufferIndex = codec.dequeueOutputBuffer(bufferInfo, 0);
while (outputBufferIndex >= 0) {
ByteBuffer outputBuffer = codecOutputBuffers[outputBufferIndex];
byte[] outData = new byte[bufferInfo.size];
outputBuffer.get(outData);
fos.write(outData);
codec.releaseOutputBuffer(outputBufferIndex, false);
outputBufferIndex = codec.dequeueOutputBuffer(bufferInfo, 0);
}
```
这个代码片段中,我们从编码器的输出缓冲区中获取编码后的数据(如果没有可用的,就会一直等待),将数据写入文件中,然后将缓冲区释放。
6. 停止编码器并释放资源:
```
codec.stop();
codec.release();
fos.flush();
fos.close();
```
这个代码片段中,我们停止编码器并释放它占用的资源,同时将输出流刷新并关闭。
以上就是使用 MediaCodec 进行安卓 PCM 转 MP3 的大致步骤,你可以根据自己的实际需求进行代码实现。
java faac pcm转aac
### 回答1:
Java是一种广泛应用于软件开发中的编程语言,而FAAC是一款常用的开源编码器,可以将PCM(脉冲编码调制)格式的音频转换成AAC(高级音频编码)格式。下面是一种使用Java和FAAC库进行PCM转AAC的简单示例。
首先,需要在Java项目中引入FAAC库的依赖。可以通过Maven等构建工具来添加FAAC库的依赖项。然后,在Java代码中调用FAAC库提供的方法来实现PCM转AAC的功能。
```java
import com.googlecode.mp4parser.authoring.Sample;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.nio.ByteBuffer;
import java.nio.ByteOrder;
import java.nio.ShortBuffer;
import java.util.ArrayList;
public class PCM2AACConverter {
// PCM转AAC的方法
public static void pcmToAac(String inputPath, String outputPath) {
try {
FileInputStream fis = new FileInputStream(inputPath);
FileOutputStream fos = new FileOutputStream(outputPath);
int channels = 2; // 音频通道数
int sampleRate = 44100; // 采样率
int bitRate = 128000; // 码率
// 创建FAAC编码器
com.googlecode.mp4parser.authoring.Sample sample = new com.googlecode.mp4parser.authoring.Sample();
sample.begin(channels, sampleRate, bitRate);
byte[] data = new byte[1024];
int bytesRead = 0;
while ((bytesRead = fis.read(data)) > 0) {
// 将PCM数据转换成short类型的数组
ShortBuffer shortBuffer = ByteBuffer.wrap(data).order(ByteOrder.LITTLE_ENDIAN).asShortBuffer();
short[] pcmData = new short[shortBuffer.remaining()];
shortBuffer.get(pcmData);
// 将PCM数据添加到FAAC编码器
sample.add(pcmData);
}
// 完成PCM数据添加操作
sample.end();
// 获取AAC编码后的数据
ArrayList<byte[]> aacData = sample.getAACData();
// 将AAC数据写入输出文件
for (byte[] aacFrame : aacData) {
fos.write(aacFrame);
}
fis.close();
fos.close();
} catch (Exception e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
String inputPath = "input.pcm"; // 输入PCM文件的路径
String outputPath = "output.aac"; // 输出AAC文件的路径
pcmToAac(inputPath, outputPath);
}
}
```
上述代码使用了FAAC库提供的`Sample`类来进行PCM转AAC操作。通过`pcmToAac`方法,将输入的PCM文件转换成AAC格式并输出到指定路径。在此过程中,需要指定音频的通道数、采样率和码率等参数。
要使用上述方法,首先需要确保Java项目已经引入FAAC库的依赖,同时将输入的PCM文件和输出的AAC文件放置在正确的路径下。
以上是一个简单的示例,仅供参考。实际应用中,可能需要根据具体需求对代码进行修改和优化。
### 回答2:
Java FAAC PCM转AAC可以通过使用FAAC库和Java编程语言来实现。以下是一个简单的示例代码来演示如何使用Java FAAC库将PCM音频文件转换为AAC格式:
```java
import com.googlecode.faac.Faac;
import com.googlecode.faac.FaacConfig;
import com.googlecode.faac.FaacConfigParams;
import com.googlecode.faac.FaacInstance;
public class PCMtoAACConverter {
public static void main(String[] args) {
String pcmFile = "input.pcm";
String aacFile = "output.aac";
// 初始化FAAC库
Faac faac = Faac.getInstance();
// 创建FAAC实例
FaacInstance faacInstance = faac.faacEncOpenDefault(48000, 2);
// 设置FAAC配置参数
FaacConfig faacConfig = faac.faacEncGetCurrentConfiguration(faacInstance);
FaacConfigParams configParams = new FaacConfigParams();
configParams.setBitrate(128000);
configParams.setInputFormat(Faac.FAAC_INPUT_FORMAT_16BIT);
faacConfig.setParams(configParams);
faac.faacEncSetConfiguration(faacInstance, faacConfig);
// 打开PCM文件和AAC文件
faac.faacEncOpenFile(faacInstance, pcmFile, aacFile);
// 开始转换
faac.faacEncEncode(faacInstance);
// 关闭文件和FAAC实例
faac.faacEncClose(faacInstance);
faac.faacEncCloseFile(faacInstance);
System.out.println("PCM转AAC完成!");
}
}
```
上述代码示例使用了FAAC库提供的相关函数来实现PCM文件转换为AAC文件的功能。其中,需先准备好待转换的PCM音频文件和指定的输出AAC文件路径。代码中的配置参数可根据需求进行调整,例如比特率、通道数等。
需要注意的是,使用FAAC库需要先下载并导入合适的FAAC库文件到项目中,并进行相关的配置。此外,FAAC库是一个开源的第三方库,使用时需了解其许可证限制和相关文档。
### 回答3:
要使用Java将PCM音频文件转换为AAC格式,可以使用FAAC库。FAAC是一个用于将音频文件编码为AAC格式的开源库。
首先,需要确保已经下载并安装了FAAC库。然后,可以在Java项目中使用JNI(Java Native Interface)来调用FAAC库的功能。
在Java中使用JNI调用FAAC库时,可以使用JNIWrapper库。这个库提供了一种方便的方式来与C/C++代码进行交互。
首先,需要编写一个调用FAAC的Java类。在这个类中,可以使用JNIWrapper库提供的函数来加载FAAC库和调用它的函数。可以编写一个Java方法来将PCM文件转换为AAC文件。
示例代码如下:
```java
import com.jniwrapper.*;
import com.jniwrapper.win32.jni.*;
import com.jniwrapper.util.*;
public class FaacConverter {
private static final String LIBRARY_NAME = "your_FAAC_library_name";
static {
NativeLibraryLoader.loadLibrary(LIBRARY_NAME);
}
public static native int convertPcmToAac(String pcmFilePath, String aacFilePath);
public static void main(String[] args) {
String pcmFilePath = "/path/to/pcm/file.pcm";
String aacFilePath = "/path/to/aac/file.aac";
int result = convertPcmToAac(pcmFilePath, aacFilePath);
if (result == 0) {
System.out.println("PCM file converted to AAC successfully.");
} else {
System.out.println("Failed to convert PCM file to AAC.");
}
}
}
```
要使用这个示例代码,需要将FAAC库文件与Java项目一起编译,并将LIBRARY_NAME变量设置为FAAC库的名称。
编写完Java类后,可以使用Java编译器将其编译为可执行的Java程序。
然后,可以通过运行这个程序来将PCM文件转换为AAC文件。在main方法中,可以设置PCM文件的路径和要生成的AAC文件的路径。
运行这个程序后,如果转换成功,将会在控制台打印出"PCM file converted to AAC successfully."的消息,否则将会打印出"Failed to convert PCM file to AAC."的消息。
这就是使用Java将PCM音频文件转换为AAC格式的基本步骤。希望对你有所帮助!
阅读全文
相关推荐
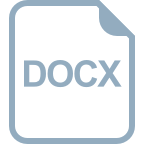
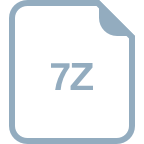
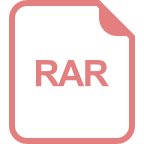
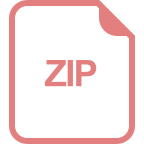
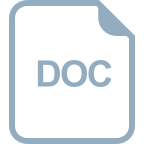
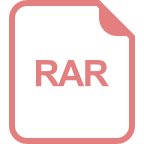
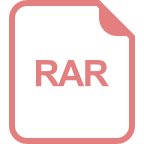
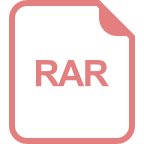
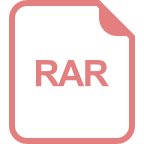
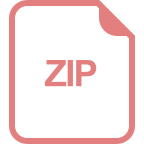
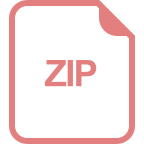
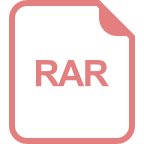
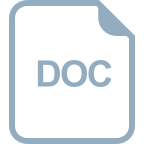
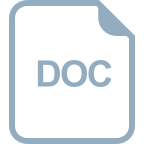