mysql中怎么把NEW直接转为json
时间: 2024-03-27 11:39:20 浏览: 12
在 MySQL 中,可以使用 JSON_OBJECT() 函数将数据转换为 JSON 格式,但是需要手动指定每一个键和值。如果要将整个行转换为 JSON 格式,可以使用 JSON_OBJECTAGG() 函数。
例如,假设我们有一个名为 `users` 的表,其中包含 `id`、`name` 和 `age` 三个字段。要将整个行转换为 JSON 格式,可以使用以下查询:
```
SELECT JSON_OBJECTAGG(column_name, column_value) AS json_data
FROM (
SELECT 'id', NEW.id AS id, 'name', NEW.name AS name, 'age', NEW.age AS age
) t;
```
这将返回一个包含所有行数据的 JSON 对象,其中键为字段名,值为对应的值。你可以将 `column_name` 替换为你想要的键名。
相关问题
mysql中直接使用NEW转json
在MySQL中,可以使用`JSON_OBJECT()`函数将数据转换为JSON格式,但它需要手动指定每一个键和值。如果要将整个行转换为JSON格式,可以使用`JSON_OBJECTAGG()`函数。
例如,假设我们有一个名为`users`的表,其中包含`id`、`name`和`age`三个字段。要将整个行转换为JSON格式,可以使用以下查询:
```
SELECT JSON_OBJECTAGG(column_name, column_value) AS json_data
FROM (
SELECT 'id', id FROM users UNION ALL
SELECT 'name', name FROM users UNION ALL
SELECT 'age', age FROM users
) t;
```
这将返回一个包含所有行数据的JSON对象。你可以将`column_name`替换为你想要的键名。
MYSQL中OLD直接转json字符串怎么解决
在MySQL中,如果你需要将一个旧的JSON格式的字符串(使用反斜杠转义双引号)转换成新的JSON格式,可以使用JSON_OBJECT()和JSON_EXTRACT()函数来实现。例如:
```sql
SELECT JSON_OBJECT('name', JSON_EXTRACT('{"name": "John", "age": 30, "city": "New York"}', '$.name'),
'age', JSON_EXTRACT('{"name": "John", "age": 30, "city": "New York"}', '$.age'),
'city', JSON_EXTRACT('{"name": "John", "age": 30, "city": "New York"}', '$.city')
) AS json;
```
这将输出以下JSON字符串:
```
{"name": "John", "age": 30, "city": "New York"}
```
请注意,JSON_EXTRACT()函数中的第一个参数应该是旧的JSON格式的字符串,第二个参数应该是你要提取的JSON键。JSON_OBJECT()函数中的每个参数都应该是键值对,其中键是新的JSON格式的键,值是JSON_EXTRACT()函数的结果。
如果你需要将新的JSON格式的字符串转换回旧的JSON格式,可以使用JSON_QUOTE()函数来转义双引号。例如:
```sql
SELECT REPLACE(JSON_QUOTE('{"name": "John", "age": 30, "city": "New York"}'), '\\"', '"') AS old_json;
```
这将输出以下JSON字符串:
```
{"name": "John", "age": 30, "city": "New York"}
```
在这个例子中,JSON_QUOTE()函数将新的JSON格式的字符串转义为旧的JSON格式的字符串,然后使用REPLACE()函数去掉转义的双引号。
相关推荐
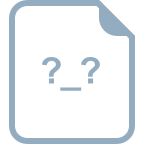
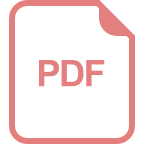












