你能帮我用python写一个连接kinect2设备的程序吗
时间: 2023-08-31 08:25:50 浏览: 185
### 回答1:
是的,我可以帮助您编写一个使用 Python 连接 Kinect 2 设备的程序。不过,请先确保您已经安装了相关的驱动程序和开发工具包(如 libfreenect2),并且确保您的设备已经正常连接到电脑上。
如果您需要代码示例,可以参考以下内容:
```
import freenect
import cv2
import numpy as np
def get_depth():
return freenect.sync_get_depth()[0]
def get_video():
return freenect.sync_get_video()[0]
while True:
depth = get_depth()
video = get_video()
depth = 255 * np.logical_and(depth >= 10, depth <= 2000) / 2000
depth = depth.astype(np.uint8)
video = cv2.cvtColor(video, cv2.COLOR_RGB2BGR)
cv2.imshow('Depth', depth)
cv2.imshow('Video', video)
if cv2.waitKey(10) == 27:
break
cv2.destroyAllWindows()
```
以上代码使用了 libfreenect2 库来连接 Kinect 2 设备并获取深度图像和彩色图像。希望对您有所帮助!
### 回答2:
当然可以。连接Kinect2设备的程序通常使用Microsoft提供的开发工具包(SDK)。在Python中,可以使用PyKinect2库来访问Kinect2设备功能。
首先,确保你的计算机上已经安装了Kinect2设备所需要的驱动程序。然后,你需要安装PyKinect2库。你可以使用pip工具在命令行中运行以下命令来安装PyKinect2:
pip install pykinect2
安装完成后,你可以开始编写连接Kinect2设备的程序。下面是一个简单的示例:
```python
import ctypes
import _ctypes
# 导入PyKinect2库
from pykinect2 import PyKinectV2
from pykinect2.PyKinectV2 import *
# 创建Kinect2设备对象
kinect = PyKinectV2()
# 初始化Kinect2
kinect.init()
# 设置要读取的数据类型
kinect.set_color_frame_type(ColorFrameType.ColorFrameType_Color)
# 创建一个窗口用于显示Kinect2捕获的图像
window = ctypes.windll.user32.FindWindowW(None, ctypes.cast('Kinect2', ctypes.c_wchar_p))
# 循环读取并处理Kinect2的数据
while True:
# 读取彩色帧
frame = kinect.get_last_color_frame()
# 显示彩色帧
ctypes.windll.user32.SetForegroundWindow(window)
# 释放帧资源
frame = None
# 检查是否传输退出命令
if ctypes.windll.user32.PeekMessageW(None, 0, 0, 0, 1):
break
# 关闭Kinect2设备
kinect.close()
```
这个示例程序会初始化Kinect2设备并读取彩色帧数据。你可以修改代码来读取其他类型的数据,如深度数据、骨骼追踪等。
请注意,这只是一个基本的示例,你可以按照自己的需求进行更多的定制和扩展。希望这可以帮到你!
### 回答3:
当然可以帮你编写一个连接Kinect2设备的Python程序。
首先,你需要确保你的计算机上安装了Kinect2设备的驱动程序和Kinect2软件开发工具包(SDK)。
接下来,你可以使用Python中的一个开源库`pykinect2`来连接和操作Kinect2设备。你可以通过`pip`命令安装它:`pip install pykinect2`。
下面是一个简单的示例代码,展示了如何使用Python连接Kinect2设备并获取它的彩色图像和深度图像:
```python
import cv2
from pykinect2 import PyKinectV2, PyKinectRuntime
# 初始化Kinect2设备
kinect = PyKinectRuntime.PyKinectRuntime(PyKinectV2.FrameSourceTypes_Color | PyKinectV2.FrameSourceTypes_Depth)
# 创建窗口并设置窗口标题
cv2.namedWindow('Kinect2', cv2.WINDOW_NORMAL)
cv2.setWindowTitle('Kinect2', 'Kinect2')
# 进入主循环
while True:
# 检查Kinect2设备是否准备好
if kinect.has_new_color_frame() and kinect.has_new_depth_frame():
# 获取彩色图像
color_frame = kinect.get_last_color_frame()
color_image = color_frame.reshape((kinect.color_frame_desc.Height, kinect.color_frame_desc.Width, 4))
# 获取深度图像
depth_frame = kinect.get_last_depth_frame()
depth_image = depth_frame.reshape((kinect.depth_frame_desc.Height, kinect.depth_frame_desc.Width))
# 显示彩色图像和深度图像
cv2.imshow('Kinect2', color_image)
cv2.imshow('Depth', depth_image)
# 检测键盘输入,按下Esc键退出程序
if cv2.waitKey(1) == 27:
break
# 释放资源
kinect.close()
cv2.destroyAllWindows()
```
上述代码首先导入了`cv2`和`pykinect2`模块,然后创建了一个`PyKinectRuntime`对象来连接Kinect2设备。在主循环中,我们检查是否有新的彩色图像和深度图像生成,并使用`get_last_color_frame()`和`get_last_depth_frame()`函数获取相应的帧数据。最后,使用`cv2.imshow()`函数显示彩色图像和深度图像,并使用`cv2.waitKey()`函数检测键盘输入。
希望这个示例能帮助你开始编写连接Kinect2设备的Python程序!如有其他问题,请随时向我提问。
阅读全文
相关推荐
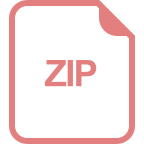

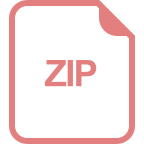
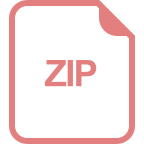
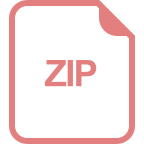
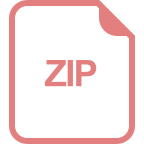
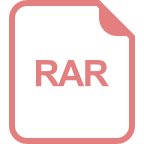
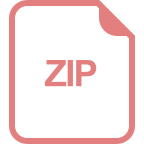
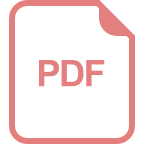
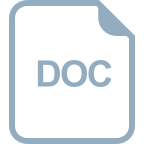
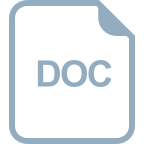
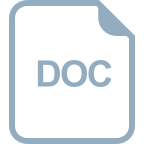
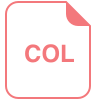



