linux c 代码实现netlink检测所有网口up与down
时间: 2024-05-11 11:19:04 浏览: 217
下面是一个简单的示例代码,用于检测所有网口的 UP 和 DOWN 状态。该代码使用了 Netlink Socket API,需要在 Linux 系统中编译和运行。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/socket.h>
#include <linux/netlink.h>
#include <linux/rtnetlink.h>
#define BUFSIZE 8192
int main() {
int fd;
struct sockaddr_nl sa;
char buf[BUFSIZE];
struct nlmsghdr *nh;
struct ifinfomsg *ifinfo;
int len;
// 创建 Netlink Socket
if ((fd = socket(AF_NETLINK, SOCK_RAW, NETLINK_ROUTE)) < 0) {
perror("socket");
exit(1);
}
memset(&sa, 0, sizeof(sa));
sa.nl_family = AF_NETLINK;
sa.nl_groups = RTMGRP_LINK; // 监听网卡变化事件
if (bind(fd, (struct sockaddr *)&sa, sizeof(sa)) < 0) {
perror("bind");
exit(1);
}
// 接收 Netlink 消息
while ((len = recv(fd, buf, BUFSIZE, 0)) > 0) {
for (nh = (struct nlmsghdr *)buf; NLMSG_OK(nh, len); nh = NLMSG_NEXT(nh, len)) {
if (nh->nlmsg_type == NLMSG_DONE) {
break;
} else if (nh->nlmsg_type == NLMSG_ERROR) {
perror("recv");
exit(1);
} else if (nh->nlmsg_type != RTM_NEWLINK) {
continue;
}
ifinfo = (struct ifinfomsg *)NLMSG_DATA(nh);
// 监听到网卡 UP 或 DOWN 事件
if (ifinfo->ifi_change & (IFACE_UP | IFACE_DOWN)) {
printf("Interface %d is %s\n", ifinfo->ifi_index,
(ifinfo->ifi_flags & IFF_UP) ? "UP" : "DOWN");
}
}
}
close(fd);
return 0;
}
```
该程序使用了 Netlink Socket API 监听 RTMGRP_LINK 组,对于每个 RTM_NEWLINK 消息,解析其中的 ifinfomsg 结构体,检查其 ifi_change 和 ifi_flags 成员以确定网卡 UP 或 DOWN 事件。
阅读全文
相关推荐
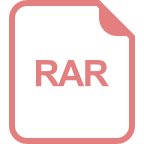
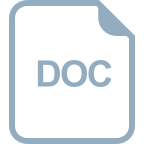
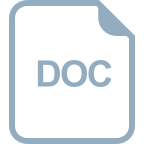
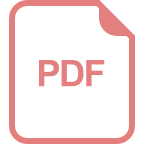
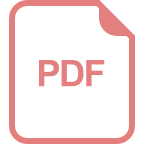
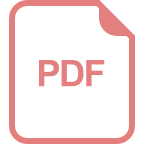
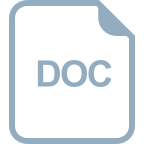
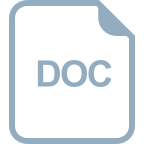
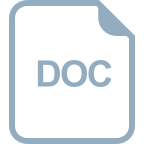
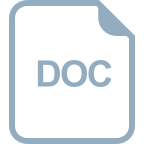
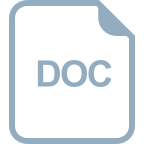
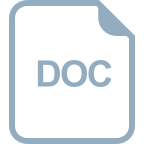
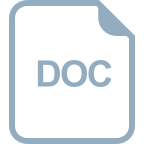