c# 调用c++dll
时间: 2023-07-29 12:08:04 浏览: 72
在 C# 中调用 C++ DLL 的步骤如下:
1. 在 C++ 中定义一个 DLL 导出函数,该函数需要使用 extern "C" 声明,并使用 __declspec(dllexport) 修饰符导出。该函数的参数和返回值类型需要与 C# 中的声明一致。
2. 在 C# 中声明 DLLImport 属性,用于指定 C++ DLL 的名称和函数签名。
3. 在 C# 中调用 C++ DLL 中的函数。
下面是一个简单的示例,演示如何在 C# 中调用 C++ DLL:
C++ DLL 代码:
```cpp
// example.cpp
#include "stdafx.h"
extern "C" __declspec(dllexport) int add(int a, int b)
{
return a + b;
}
```
C# 代码:
```csharp
using System.Runtime.InteropServices;
class Program
{
[DllImport("example.dll", CallingConvention = CallingConvention.Cdecl)]
public static extern int add(int a, int b);
static void Main(string[] args)
{
int result = add(1, 2);
System.Console.WriteLine(result);
}
}
```
在上面的示例中,我们首先在 C++ DLL 中定义了一个名为 add 的函数,并使用 __declspec(dllexport) 修饰符导出。然后我们在 C# 中使用 DllImport 属性指定了 example.dll 的名称和 add 函数的签名。最后,我们在 Main 函数中调用了 add 函数,并将结果打印到控制台上。
需要注意的是,在使用 C++ DLL 时,需要注意函数的调用约定。C++ 默认使用的是 __cdecl 调用约定,而 C# 默认使用的是 __stdcall 调用约定。因此,在使用 C++ DLL 时,需要使用 CallingConvention 属性指定函数的调用约定,以免出现调用错误的情况。
相关推荐
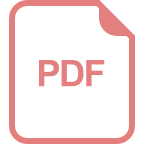















