java使用snakeyaml生成gpload的control配置文件,需要java语言实现,要求用到build设计模式,配置文件内容模型化并且包含VERSION、DATABASE、USER、HOST、PORT、GPLOAD、INPUT、EXTERNAL、OUTPUT
时间: 2023-09-28 15:03:22 浏览: 100
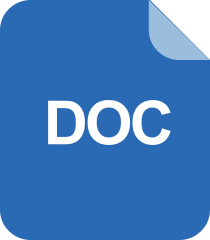
java配置文件,用于安装java
以下是一个可能的实现,使用了builder设计模式,将配置文件内容模型化为一个Config类:
```java
public class Config {
private final String version;
private final String database;
private final String user;
private final String host;
private final int port;
private final Gpload gpload;
private final Input input;
private final External external;
private final Output output;
private Config(ConfigBuilder builder) {
this.version = builder.version;
this.database = builder.database;
this.user = builder.user;
this.host = builder.host;
this.port = builder.port;
this.gpload = builder.gpload;
this.input = builder.input;
this.external = builder.external;
this.output = builder.output;
}
public String getVersion() {
return version;
}
public String getDatabase() {
return database;
}
public String getUser() {
return user;
}
public String getHost() {
return host;
}
public int getPort() {
return port;
}
public Gpload getGpload() {
return gpload;
}
public Input getInput() {
return input;
}
public External getExternal() {
return external;
}
public Output getOutput() {
return output;
}
public static class ConfigBuilder {
private String version;
private String database;
private String user;
private String host;
private int port;
private Gpload gpload;
private Input input;
private External external;
private Output output;
public ConfigBuilder version(String version) {
this.version = version;
return this;
}
public ConfigBuilder database(String database) {
this.database = database;
return this;
}
public ConfigBuilder user(String user) {
this.user = user;
return this;
}
public ConfigBuilder host(String host) {
this.host = host;
return this;
}
public ConfigBuilder port(int port) {
this.port = port;
return this;
}
public ConfigBuilder gpload(Gpload gpload) {
this.gpload = gpload;
return this;
}
public ConfigBuilder input(Input input) {
this.input = input;
return this;
}
public ConfigBuilder external(External external) {
this.external = external;
return this;
}
public ConfigBuilder output(Output output) {
this.output = output;
return this;
}
public Config build() {
return new Config(this);
}
}
public static class Gpload {
private final String input;
private final String format;
private final String delimiter;
private final String quote;
private final String errorLimit;
private final String logErrors;
private final String tableName;
public Gpload(String input, String format, String delimiter, String quote, String errorLimit, String logErrors, String tableName) {
this.input = input;
this.format = format;
this.delimiter = delimiter;
this.quote = quote;
this.errorLimit = errorLimit;
this.logErrors = logErrors;
this.tableName = tableName;
}
public String getInput() {
return input;
}
public String getFormat() {
return format;
}
public String getDelimiter() {
return delimiter;
}
public String getQuote() {
return quote;
}
public String getErrorLimit() {
return errorLimit;
}
public String getLogErrors() {
return logErrors;
}
public String getTableName() {
return tableName;
}
}
public static class Input {
private final String type;
private final String path;
public Input(String type, String path) {
this.type = type;
this.path = path;
}
public String getType() {
return type;
}
public String getPath() {
return path;
}
}
public static class External {
private final String protocol;
private final String host;
private final int port;
public External(String protocol, String host, int port) {
this.protocol = protocol;
this.host = host;
this.port = port;
}
public String getProtocol() {
return protocol;
}
public String getHost() {
return host;
}
public int getPort() {
return port;
}
}
public static class Output {
private final String type;
private final String tableName;
public Output(String type, String tableName) {
this.type = type;
this.tableName = tableName;
}
public String getType() {
return type;
}
public String getTableName() {
return tableName;
}
}
}
```
可以使用SnakeYaml来生成配置文件:
```java
public class ControlFileGenerator {
public static void main(String[] args) throws IOException {
Config config = new Config.ConfigBuilder()
.version("1.0")
.database("mydb")
.user("myuser")
.host("localhost")
.port(5432)
.gpload(new Config.Gpload("data.csv", "csv", ",", "\"", "100", "true", "mytable"))
.input(new Config.Input("file", "/path/to/data.csv"))
.external(new Config.External("http", "example.com", 8080))
.output(new Config.Output("table", "mytable"))
.build();
Yaml yaml = new Yaml();
String output = yaml.dumpAsMap(config);
System.out.println(output);
}
}
```
输出的内容类似于:
```
database: mydb
external:
host: example.com
port: 8080
protocol: http
gpload:
delimiter: ','
errorLimit: '100'
format: csv
input: data.csv
logErrors: 'true'
quote: '"'
tableName: mytable
host: localhost
input:
path: /path/to/data.csv
type: file
output:
tableName: mytable
type: table
port: 5432
user: myuser
version: '1.0'
```
阅读全文
相关推荐
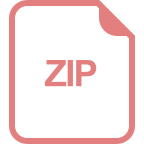
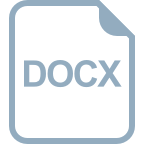
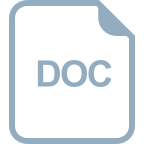
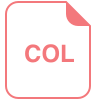
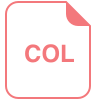
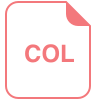
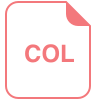
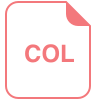
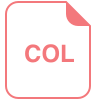
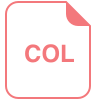
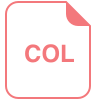
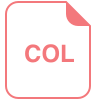
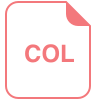
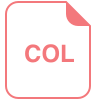
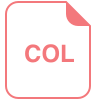
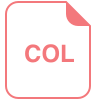
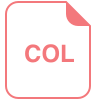
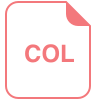