先定义一个将输入图像转换为二值图并进行边缘拟合,然后按行扫描边缘二值图像,将左右的边缘点记录下来并计算两者之间的中点位置并将中点存储下来的函数,然后定义一个利用中点拟合曲线线段并在原图上显示的函数
时间: 2023-11-22 12:53:18 浏览: 24
好的,我可以帮你完成这个任务。以下是一个用 Python 实现的示例代码:
```python
import cv2
import numpy as np
def edge_detection(image):
# Convert to grayscale
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# Apply edge detection using Canny algorithm
edges = cv2.Canny(gray, 100, 200)
# Dilate the edges to make them thicker
kernel = np.ones((3, 3), np.uint8)
edges = cv2.dilate(edges, kernel, iterations=1)
return edges
def find_edge_points(edges):
# Initialize variables
left_points = []
right_points = []
# Loop through each row in the image
for y in range(edges.shape[0]):
# Find the first nonzero pixel from the left
left_x = np.argmax(edges[y, :])
if edges[y, left_x] != 0:
left_points.append((left_x, y))
# Find the first nonzero pixel from the right
right_x = np.argmax(np.flip(edges[y, :], axis=0))
if edges[y, -right_x-1] != 0:
right_points.append((edges.shape[1]-right_x-1, y))
# Calculate the midpoint of each edge segment
midpoints = []
for i in range(len(left_points)):
x = (left_points[i][0] + right_points[i][0]) // 2
y = (left_points[i][1] + right_points[i][1]) // 2
midpoints.append((x, y))
return midpoints
def fit_curve(image, midpoints):
# Fit a polynomial curve to the midpoints
curve = np.polyfit([p[1] for p in midpoints], [p[0] for p in midpoints], 2)
# Generate points along the curve
curve_points = []
for y in range(image.shape[0]):
x = int(curve[0] * y**2 + curve[1] * y + curve[2])
if x < 0:
x = 0
elif x >= image.shape[1]:
x = image.shape[1] - 1
curve_points.append((x, y))
# Draw the curve on the original image
output = image.copy()
for i in range(len(curve_points)-1):
cv2.line(output, curve_points[i], curve_points[i+1], (0, 0, 255), 2)
return output
# Load an image
image = cv2.imread('example.jpg')
# Perform edge detection
edges = edge_detection(image)
# Find edge points and midpoints
midpoints = find_edge_points(edges)
# Fit a curve to the midpoints and draw it on the original image
output = fit_curve(image, midpoints)
# Display the result
cv2.imshow('Output', output)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这个函数将输入图像转换为二值图并进行边缘拟合,然后按行扫描边缘二值图像,将左右的边缘点记录下来并计算两者之间的中点位置并将中点存储下来。最后,它会利用中点拟合曲线线段并在原图上显示。你可以将其保存为一个 Python 文件并运行以测试它的表现。
相关推荐
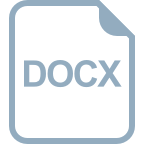
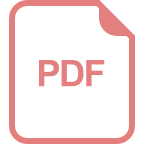
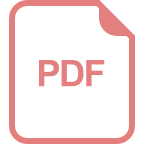











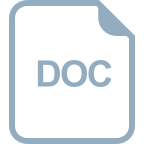
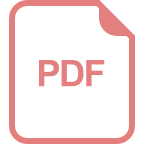
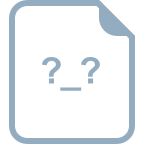
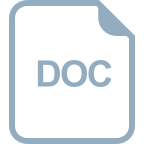