aes解密报错 Given final block not properly padded. Such issues can arise if a bad key is used during decryption,java代码如何解决,加密时候的填充模式是aes
时间: 2024-07-07 09:00:26 浏览: 169
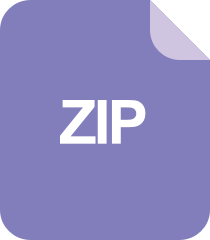
java AES加密 解决加密过长非法异常问题
AES (Advanced Encryption Standard) 解密时出现 "final block not properly padded" 错误通常是因为数据在加密后没有按照规定的填充模式正确处理。AES 加密过程中,如果数据块长度不是16字节的倍数,就需要进行填充以达到块大小。常见的填充模式有 PKCS7,其中会在剩余空间中填充1到16个字节的0,最后一位会是填充的字节数。
当解密时,如果接收到的最终块没有按预期的填充规则,就会抛出这个错误。在Java中,使用Bouncy Castle或Java Cryptography Extension (JCE) 库进行AES解密时,可以遇到这种情况。
以下是一个简单的例子,展示了如何处理这个错误:
```java
import org.bouncycastle.jce.provider.BouncyCastleProvider;
import javax.crypto.Cipher;
import javax.crypto.spec.SecretKeySpec;
import java.security.Key;
import java.security.Security;
import java.util.Base64;
Security.addProvider(new BouncyCastleProvider());
public class AESDecryption {
private static final String KEY = "your_secret_key"; // 用实际密钥替换
public static void main(String[] args) {
try {
String encryptedData = "base64_encoded_encrypted_data"; // 假设是从Base64解码得到的
byte[] keyBytes = KEY.getBytes();
Key key = new SecretKeySpec(keyBytes, "AES");
Cipher cipher = Cipher.getInstance("AES/CBC/PKCS5Padding", "BC");
cipher.init(Cipher.DECRYPT_MODE, key);
byte[] iv = getIvFromSomewhere(); // 获取CBC模式的初始化向量
cipher.updateAAD(new byte[] {0x01}); // 有时候可能需要提供额外的认证数据,这取决于加密时的情况
byte[] decryptedData = cipher.doFinal(Base64.getDecoder().decode(encryptedData));
if (cipher.getOutputSize(decryptedData.length) == decryptedData.length) { // 检查是否是正确的解密结果
System.out.println("Decrypted data: " + new String(decryptedData));
} else {
throw new RuntimeException("Invalid padding or final block error");
}
} catch (Exception e) {
e.printStackTrace();
// 这里捕获并处理特定的PaddingException,因为可能是由于填充错误
if (e instanceof PaddingException) {
throw new RuntimeException("Final block not properly padded: " + e.getMessage());
} else {
throw e;
}
}
}
private static byte[] getIvFromSomewhere() {
// 实现从存储或上下文中获取初始化向量的方法
// 假设iv已知
return new byte[] {0x00, 0x01, ...};
}
}
```
确保在使用`Cipher.updateAAD`或`Cipher.getIV`之前,你已经正确地获取了初始化向量(IV)和任何附加的认证数据(如`Cipher.updateAAD`中的数据)。
阅读全文