通过风格迁移实现的图像去雾的代码,使用VGG19网络
时间: 2024-10-24 20:04:14 浏览: 24
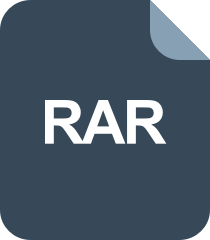
使用VGG19迁移学习实现图像风格迁移

风格迁移技术通常用于艺术创作或图像处理,其中一个应用就是去除图像中的雾气效果,让画面更加清晰。这里我们可以利用VGG19网络(Visual Geometry Group 19-layer network)来进行特征提取,然后结合内容损失和风格损失进行优化。以下是一个简单的Python代码示例,使用Keras库和TensorFlow作为后端:
```python
import tensorflow as tf
from tensorflow.keras.applications.vgg19 import VGG19, preprocess_input
from tensorflow.keras.models import Model
from tensorflow.keras.layers import Input, Dense, Lambda
# 加载预训练的VGG19模型,去掉顶层的分类层
vgg = VGG19(weights='imagenet', include_top=False)
input_img = Input(shape=(None, None, 3))
x = vgg(input_img)
# 获取中间层的输出
content_layers = ['block5_conv2'] # 内容特征层,可以根据需求调整
style_layers = ['block1_conv1', 'block2_conv1', 'block3_conv1', 'block4_conv1', 'block5_conv1'] # 风格特征层
outputs = [vgg.get_layer(name).output for name in content_layers + style_layers]
model_vgg = Model(inputs=input_img, outputs=outputs)
def gram_matrix(x):
channels = x.shape[-1]
features = tf.reshape(x, [-1, channels])
return tf.linalg.matmul(features, tf.transpose(features))
# 定义损失函数
def content_loss(base, combination):
return tf.reduce_mean(tf.square(combination - base))
def style_loss(style, combination):
S = gram_matrix(style)
C = gram_matrix(combination)
channels = S.shape[-1]
return tf.reduce_mean(tf.square(S - C) / (channels**2 * tf.size(S)))
# 假设content_image是清晰图像,style_image是带有雾气的效果图
content_weight = 1
style_weight = 1e6
combined = model_vgg(content_image)
target = model_vgg(style_image)
# 初始化生成图像
input_tensor = combined.input
combined_output = []
for layer in model_vgg.layers[:-1]:
if isinstance(layer, Lambda): # 对于Gram矩阵层,直接使用计算结果
target_feature = target[layer.name]
else:
target_feature = target[layer.output.name]
combined_output.append(Lambda(lambda x: x)(layer(combined)))
loss = content_weight * content_loss(combined_output[0], target_feature[:1]) + \
style_weight * sum([style_loss(target_feature[i:i+1], combined_output[i]) for i in range(1, len(style_layers))])
# 使用梯度下降优化器
optimizer = tf.optimizers.Adam()
input_img.set_shape(style_image.shape)
delta = tf.Variable(initial_value=tf.zeros_like(input_img), trainable=True)
grads = optimizer.gradient(loss, delta)
train_step = optimizer.apply_gradients(zip(grads, [delta]))
# 运行循环更新生成图像
for _ in range(iterations):
train_step.run()
# 最终得到去雾后的图像
result = input_img + delta.eval()
```
阅读全文
相关推荐
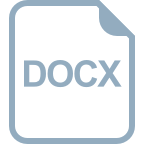















