多重if选择结构的例子
时间: 2024-01-27 07:04:10 浏览: 61
以下是一个多重if选择结构的例子:
```python
score = 85
if score >= 90:
grade = "A"
elif score >= 80:
grade = "B"
elif score >= 70:
grade = "C"
elif score >= 60:
grade = "D"
else:
grade = "F"
print("Your grade is:", grade)
```
在这个例子中,根据分数的不同,程序会根据条件选择不同的分级。如果分数大于等于90,将获得A级,如果分数大于等于80,将获得B级,以此类推。如果分数低于60,将获得F级。最后,程序会打印出相应的分级。
相关问题
综合运用嵌套if选择结构、switch选择结构、多重if选择结构实现商品换购功能
在实现商品换购功能时,综合运用嵌套 `if` 选择结构、`switch` 选择结构和多重 `if` 选择结构可以帮助我们根据不同情况做出决策。以下是简化的示例代码:
```java
public int processExchange(int oldProductID, int newProductID) {
// 嵌套if选择结构判断库存
if (checkStock(oldProductID)) {
// switch选择结构处理不同类型的商品规则
switch (oldProductID) {
case PRODUCT_A:
if (PRODUCT_A_PRICE <= calculateNewProductPrice(newProductID)) { // 新产品价格不超过旧产品的最高折扣价
return exchange(oldProductID, newProductID);
}
break;
// 其他商品类型同样处理
}
}
// 多重if选择结构处理其他特殊情况
if (!checkStock(newProductID) || isInvalidExchange(oldProductID, newProductID)) {
return -1; // 返回错误代码或处理失败的结果
}
// 正常换购流程
return exchange(oldProductID, newProductID);
}
private boolean checkStock(int productId) {
// 实现查询库存方法...
}
private int calculateNewProductPrice(int newProductID) {
// 实现计算新价格方法...
}
private int exchange(int oldProductID, int newProductID) {
// 更新库存并处理交易逻辑...
}
```
在这个例子中,嵌套的 `if` 结构用于库存检查,`switch` 则根据商品类型确定价格限制条件,而多重 `if` 可能用于处理特殊规则或者错误检查。在每个逻辑分支中,可能还需要更多的 `if` 条件来细化规则。
运用嵌套if结构和switch结构 多重if选择结构 实现商品换购功能
在JavaScript中,你可以使用多重`if`选择结构或`switch`结构来实现商品换购功能。这里我们假设有一个商品列表,每个商品都有原价、折扣价以及是否参与换购活动。以下是一个简单的例子:
```javascript
let product = {
name: 'Product A',
originalPrice: 100,
discountedPrice: null, // 可能为null表示未打折
onSale: false, // 是否参与换购
};
function checkExchange(product) {
if (product.onSale) { // 检查商品是否在换购活动
if (product.discountedPrice === null) { // 如果没有打折价格,则计算折扣价
product.discountedPrice = calculateDiscountedPrice(product.originalPrice); // 假设calculateDiscountedPrice是个函数
}
console.log(`You can exchange ${product.name} for the discounted price of $${product.discountedPrice}`);
} else {
console.log(`${product.name} is not on sale.`);
}
}
// 示例:如果商品A在换购活动中并且原价大于某个阈值,有额外优惠
function calculateDiscountedPrice(originalPrice) {
if (originalPrice > 50) {
return originalPrice * 0.8; // 给予8折优惠
} else {
return originalPrice;
}
}
checkExchange(product);
```
在这个例子中,`checkExchange`函数首先检查商品是否在换购活动中,然后根据情况决定是否计算折扣价并输出相应的信息。
如果你想用`switch`结构来实现相同的功能,可能需要转换逻辑结构,因为`switch`主要针对有限的条件分支:
```javascript
function checkExchangeWithSwitch(product) {
switch (true) {
case product.onSale && product.discountedPrice === null:
product.discountedPrice = calculateDiscountedPrice(product.originalPrice);
break;
case !product.onSale:
console.log(`${product.name} is not on sale.`);
break;
default:
console.log('Invalid condition.');
}
console.log(`You can exchange ${product.name} for the discounted price of $${product.discountedPrice}`);
}
checkExchangeWithSwitch(product);
```
但请注意,`switch`在这里并不是最佳选择,因为它不如`if...else`链更直观,特别是在处理多个复杂的逻辑条件时。
阅读全文
相关推荐
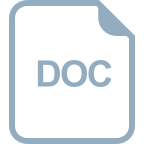
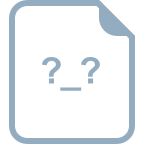
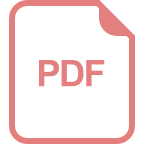
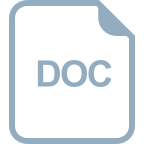
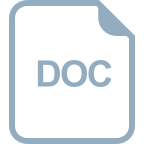
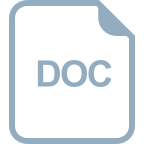
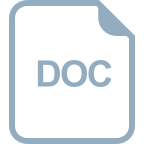
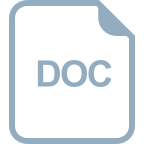
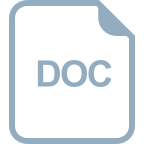
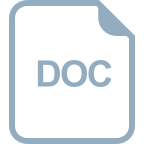
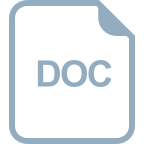
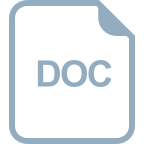
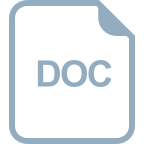
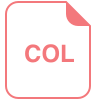


