three.js vue
时间: 2023-09-12 14:11:07 浏览: 54
Three.js 是一个用于创建 3D 图形的 JavaScript 库,而 Vue 是一个用于构建用户界面的 JavaScript 框架。它们可以一起使用,以在 Vue 应用程序中创建交互式的 3D 图形场景。
要在 Vue 中使用 Three.js,你需要先安装 Three.js 库,并在你的 Vue 组件中引入它。你可以通过 npm 或 yarn 安装 Three.js:
```
npm install three
```
或
```
yarn add three
```
然后,在你的 Vue 组件中,你可以使用 import 语句引入 Three.js:
```js
import * as THREE from 'three';
```
现在,你可以在 Vue 组件的生命周期钩子函数或其他方法中使用 Three.js 的功能来创建和渲染 3D 场景。你可以使用 Vue 的数据绑定将 Three.js 场景中的属性与 Vue 组件中的数据关联起来,以实现交互性。
例如,你可以在 Vue 组件中创建一个 Three.js 场景,并在其中添加一个立方体:
```js
export default {
data() {
return {
scene: null,
camera: null,
renderer: null,
cube: null
};
},
mounted() {
this.init();
this.animate();
},
methods: {
init() {
// 创建场景
this.scene = new THREE.Scene();
// 创建相机
this.camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 1000);
this.camera.position.z = 5;
// 创建渲染器
this.renderer = new THREE.WebGLRenderer();
this.renderer.setSize(window.innerWidth, window.innerHeight);
this.$refs.container.appendChild(this.renderer.domElement);
// 创建立方体
const geometry = new THREE.BoxGeometry();
const material = new THREE.MeshBasicMaterial({ color: 0x00ff00 });
this.cube = new THREE.Mesh(geometry, material);
this.scene.add(this.cube);
},
animate() {
requestAnimationFrame(this.animate);
this.cube.rotation.x += 0.01;
this.cube.rotation.y += 0.01;
this.renderer.render(this.scene, this.camera);
}
}
};
```
以上是一个简单的使用 Three.js 和 Vue 的示例,可以在 Vue 组件的模板中添加一个容器元素来显示 Three.js 场景:
```html
<template>
<div ref="container"></div>
</template>
```
这样,当 Vue 组件被挂载到页面上时,Three.js 场景就会被创建和渲染,并且立方体会旋转起来。
当然,这只是一个简单的示例,你可以根据需要在 Vue 组件中使用更多的 Three.js 功能来创建复杂的 3D 场景。希望这能帮到你!如果有任何问题,请随时提问。
相关推荐
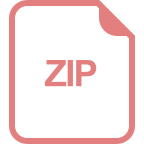
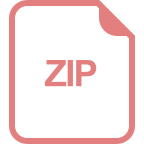
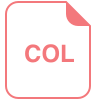
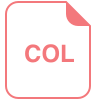
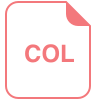
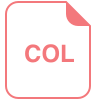
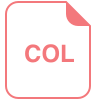










