cjson解析出来的数据如何使用
时间: 2024-04-24 17:24:19 浏览: 156
cjson是一个用于处理JSON数据的Lua库,它可以将JSON字符串解析为Lua表,或者将Lua表转换为JSON字符串。要使用cjson解析出来的数据,你可以按照以下步骤进行操作:
1. 导入cjson库:在Lua脚本中,首先需要导入cjson库,可以使用类似`local cjson = require("cjson")`的语句导入。
2. 解析JSON字符串:使用cjson库的`cjson.decode()`函数将JSON字符串解析为Lua表。例如:
```lua
local jsonStr = '{"name": "John", "age": 30}'
local jsonObj = cjson.decode(jsonStr)
```
3. 使用解析后的Lua表:解析后的数据将会被转换为Lua表,你可以像操作普通的Lua表一样使用它们。通过键名访问相应的值,例如:
```lua
local name = jsonObj.name
local age = jsonObj.age
```
4. 若需要,将Lua表转换为JSON字符串:如果需要将Lua表转换为JSON字符串,可以使用cjson库的`cjson.encode()`函数。例如:
```lua
local newJsonStr = cjson.encode(jsonObj)
```
这样,你就可以使用cjson解析出来的数据进行进一步操作或者将其转换为JSON字符串。记得根据你的具体需求来使用解析后的数据。
相关问题
如何stm32使用cjson解析串口数据
您可以先将串口数据读入缓存区,然后使用cJSON库中的函数对缓存区中的JSON格式数据进行解析。具体步骤可以参考如下代码:
```c
char json_str[256]; // 假设读入的JSON字符串最长为256字节
char buffer[1024]; // 假设缓存区大小为1024字节
// 读取串口数据到缓存区中
int n = read(serial_port, buffer, sizeof(buffer));
// 处理读取到的数据
for (int i = 0; i < n; i++) {
// 如果读到的是 '{',就表示已经读到了一个完整的JSON字符串
if (buffer[i] == '{') {
// 将JSON字符串拷贝到json_str中
strncpy(json_str, &buffer[i], sizeof(json_str));
// 解析JSON字符串
cJSON* root = cJSON_Parse(json_str);
if (root == NULL) {
printf("Error parsing JSON: %s\n", cJSON_GetErrorPtr());
} else {
// 在这里进行相应的处理
...
}
}
}
```
希望这个回答对您有帮助。如果您有其他问题,请随时问我。
cjson解析下面的数据
假设需要解析的JSON数据如下:
```
{
"name": "John",
"age": 30,
"city": "New York",
"hobbies": ["reading", "traveling", "swimming"],
"education": {
"degree": "Master",
"major": "Computer Science"
}
}
```
使用cJSON库进行解析的示例代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include "cJSON.h"
int main() {
char *json_str = "{\"name\":\"John\",\"age\":30,\"city\":\"New York\",\"hobbies\":[\"reading\",\"traveling\",\"swimming\"],\"education\":{\"degree\":\"Master\",\"major\":\"Computer Science\"}}";
cJSON *root = cJSON_Parse(json_str);
if (root == NULL) {
printf("Error before: [%s]\n", cJSON_GetErrorPtr());
return 1;
}
cJSON *name = cJSON_GetObjectItemCaseSensitive(root, "name");
if (cJSON_IsString(name) && (name->valuestring != NULL)) {
printf("Name: %s\n", name->valuestring);
}
cJSON *age = cJSON_GetObjectItemCaseSensitive(root, "age");
if (cJSON_IsNumber(age)) {
printf("Age: %d\n", age->valueint);
}
cJSON *city = cJSON_GetObjectItemCaseSensitive(root, "city");
if (cJSON_IsString(city) && (city->valuestring != NULL)) {
printf("City: %s\n", city->valuestring);
}
cJSON *hobbies = cJSON_GetObjectItemCaseSensitive(root, "hobbies");
if (cJSON_IsArray(hobbies)) {
int i, size = cJSON_GetArraySize(hobbies);
printf("Hobbies:\n");
for (i = 0; i < size; i++) {
cJSON *item = cJSON_GetArrayItem(hobbies, i);
if (cJSON_IsString(item) && (item->valuestring != NULL)) {
printf(" %s\n", item->valuestring);
}
}
}
cJSON *education = cJSON_GetObjectItemCaseSensitive(root, "education");
if (cJSON_IsObject(education)) {
cJSON *degree = cJSON_GetObjectItemCaseSensitive(education, "degree");
if (cJSON_IsString(degree) && (degree->valuestring != NULL)) {
printf("Education - Degree: %s\n", degree->valuestring);
}
cJSON *major = cJSON_GetObjectItemCaseSensitive(education, "major");
if (cJSON_IsString(major) && (major->valuestring != NULL)) {
printf("Education - Major: %s\n", major->valuestring);
}
}
cJSON_Delete(root);
return 0;
}
```
运行结果:
```
Name: John
Age: 30
City: New York
Hobbies:
reading
traveling
swimming
Education - Degree: Master
Education - Major: Computer Science
```
解析过程中,先使用cJSON_Parse函数将JSON字符串解析成一个cJSON对象(即root)。然后,使用cJSON_GetObjectItemCaseSensitive函数分别获取其中的各个成员对象,并使用不同的cJSON类型判断函数(如cJSON_IsString、cJSON_IsNumber、cJSON_IsArray等)判断其类型。最后,使用各自的value获取成员对象的值。最后,使用cJSON_Delete函数释放cJSON对象。
阅读全文
相关推荐
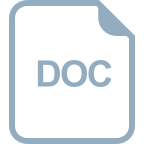
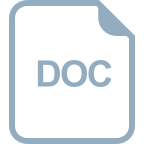
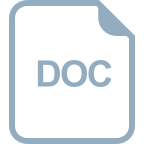
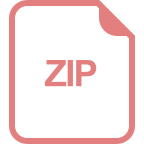
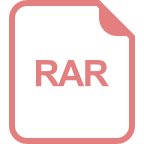
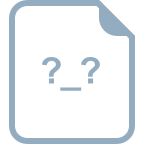
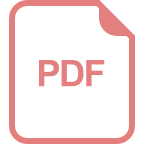
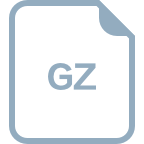
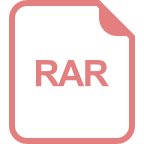
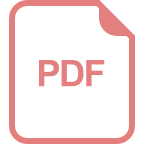
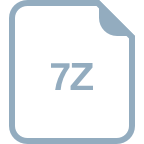
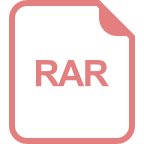




