写一个HTML代码输入姓名,评论内容,点击“评论”按钮后,将该条评论附上当前时间并添加到下方留言区; 再次输入则将新评论增加到留言区最前方,添加留言区的内容使用JS创建元素的方式完成。
时间: 2023-08-31 22:25:51 浏览: 134
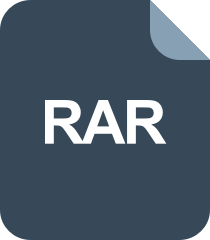
添加留言源码,可以实现留言的添加
### 回答1:
好的,这是一个关于HTML和JS的问题,我可以回答。以下是代码示例:
HTML代码部分:
```
<div>
<label for="name">姓名:</label>
<input type="text" id="name" />
</div>
<div>
<label for="comment">评论:</label>
<textarea id="comment"></textarea>
</div>
<button id="submit-btn">评论</button>
<div id="message-board"></div>
```
JS代码部分:
```
const nameInput = document.querySelector('#name')
const commentInput = document.querySelector('#comment')
const submitBtn = document.querySelector('#submit-btn')
const messageBoard = document.querySelector('#message-board')
const comments = []
submitBtn.addEventListener('click', function() {
const name = nameInput.value.trim()
const comment = commentInput.value.trim()
if (!name || !comment) {
return
}
const now = new Date()
const time = now.toLocaleString()
const newComment = {
name,
comment,
time
}
comments.unshift(newComment)
renderComments()
nameInput.value = ''
commentInput.value = ''
})
function renderComments() {
messageBoard.innerHTML = ''
comments.forEach(comment => {
const div = document.createElement('div')
const pName = document.createElement('p')
const pComment = document.createElement('p')
const pTime = document.createElement('p')
pName.textContent = `姓名:${comment.name}`
pComment.textContent = `评论:${comment.comment}`
pTime.textContent = `时间:${comment.time}`
div.appendChild(pName)
div.appendChild(pComment)
div.appendChild(pTime)
messageBoard.appendChild(div)
})
}
renderComments()
```
当用户点击“评论”按钮后,会将输入的姓名和评论内容组成对象添加到一个数组中,并使用JS创建元素的方式将最新的评论添加到留言区最前方。同时,用户再次输入评论时也会将新评论增加到留言区最前方。
### 回答2:
以下是一个实现该功能的HTML代码:
```html
<!DOCTYPE html>
<html>
<head>
<title>留言板</title>
</head>
<body>
<h1>留言板</h1>
<form id="commentForm">
<label for="name">姓名:</label>
<input type="text" id="name" required><br><br>
<label for="comment">评论内容:</label><br>
<textarea id="comment" required></textarea><br><br>
<button type="button" onclick="addComment()">评论</button>
</form>
<div id="commentSection">
<h2>留言区</h2>
<div id="comments"></div>
</div>
<script>
function addComment() {
var name = document.getElementById("name").value;
var comment = document.getElementById("comment").value;
var currentTime = new Date().toLocaleString();
var commentContainer = document.createElement("div");
commentContainer.innerHTML = "<strong>" + name + "</strong> 评论于 " + currentTime + "</br>" + comment + "</br></br>";
var commentSection = document.getElementById("comments");
commentSection.insertBefore(commentContainer, commentSection.firstChild);
document.getElementById("commentForm").reset();
}
</script>
</body>
</html>
```
这段代码实现了一个简单的留言板功能。通过HTML表单输入姓名和评论内容,点击"评论"按钮后会调用`addComment`函数。该函数获取输入的姓名、评论内容和当前时间,然后创建一个包含姓名、时间和评论内容的`<div>`元素,并将它插入到留言区的最前方。最后,清空表单以方便下一次输入。
### 回答3:
以下是一个简单的HTML代码示例,实现了根据用户输入的姓名和评论内容,点击评论按钮后将评论附上当前时间并添加到留言区,并且新评论会在最前方添加。
```html
<!DOCTYPE html>
<html>
<head>
<title>留言板</title>
<style>
#comment-section {
margin-top: 20px;
border: 1px solid #ccc;
padding: 10px;
}
</style>
</head>
<body>
<div>
<input type="text" id="name-input" placeholder="请输入姓名">
<input type="text" id="comment-input" placeholder="请输入评论内容">
<button onclick="addComment()">评论</button>
</div>
<div id="comment-section">
</div>
<script>
function addComment() {
var name = document.getElementById("name-input").value;
var comment = document.getElementById("comment-input").value;
var date = new Date();
var commentSection = document.getElementById("comment-section");
var newComment = document.createElement("div");
var commentContent = document.createTextNode(name + ":" + comment + "(" + date.toLocaleString() + ")");
newComment.appendChild(commentContent);
commentSection.insertBefore(newComment, commentSection.firstChild);
document.getElementById("name-input").value = "";
document.getElementById("comment-input").value = "";
}
</script>
</body>
</html>
```
使用上述HTML代码,用户可以在姓名输入框和评论内容输入框中输入信息,然后点击"评论"按钮即可将评论附上当前时间并添加到留言区。新评论会在最前方添加。
阅读全文
相关推荐
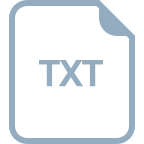
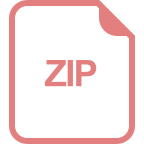
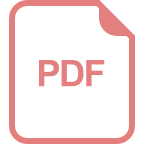
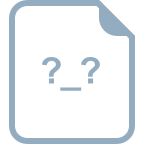
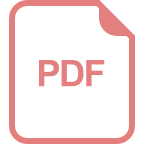
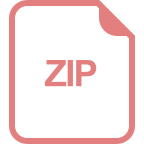
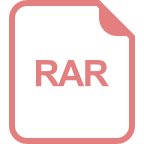
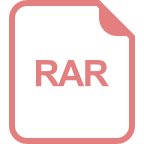
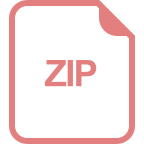
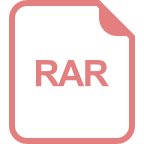
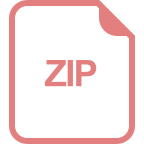
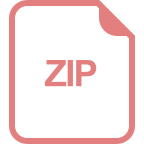
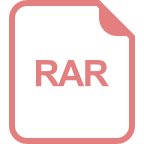
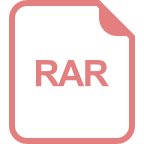