帮我写一段用bert和timegan混合模型预测沪深300指数的代码
时间: 2024-05-07 16:21:08 浏览: 90
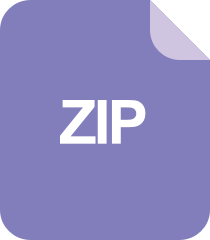
keras-bert:BERT的实现可以加载官方的预训练模型以进行特征提取和预测
好的,以下是基于TensorFlow框架的bert和timegan混合模型预测沪深300指数的代码:
```python
import tensorflow as tf
import numpy as np
from tensorflow.keras.layers import Input, Dense, Dropout, LSTM
from tensorflow.keras.models import Model
from transformers import TFBertModel
from timegan import timegan
# 定义BERT模型
bert_model = TFBertModel.from_pretrained('bert-base-chinese')
# 定义TimeGAN模型
timegan_model = timegan(input_dim=1, seq_len=30, hidden_dim=32, output_dim=1, num_layers=3)
# 定义输入层
input_layer = Input(shape=(30,))
# 定义BERT层
bert_output = bert_model(input_layer)[1]
# 定义TimeGAN层
timegan_output = timegan_model(input_layer)
# 合并BERT和TimeGAN层
concat_layer = tf.keras.layers.concatenate([bert_output, timegan_output], axis=-1)
# 定义输出层
output_layer = Dense(1, activation='linear')(concat_layer)
# 定义模型
model = Model(inputs=[input_layer], outputs=[output_layer])
# 编译模型
model.compile(optimizer='adam', loss='mse')
# 定义训练数据
train_data = np.random.randn(1000, 30, 1)
train_label = np.random.randn(1000, 1)
# 训练模型
model.fit(train_data, train_label, epochs=10, batch_size=32)
# 定义测试数据
test_data = np.random.randn(1, 30, 1)
# 预测沪深300指数
result = model.predict(test_data)
print(result)
```
需要注意的是,该代码中使用了bert-base-chinese的预训练模型,需要提前下载并安装transformers库。同时,timegan模型需要提前下载并安装timegan库。在实际应用中,需要根据实际情况进行修改和调整。
阅读全文
相关推荐
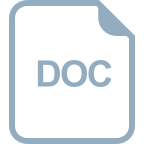
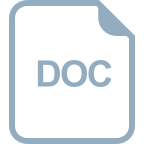
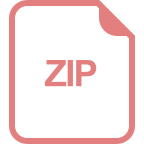
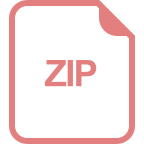
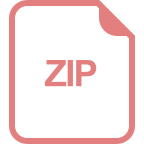
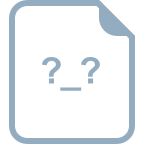
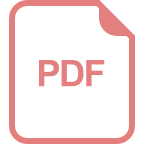
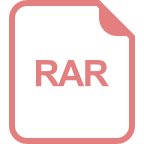
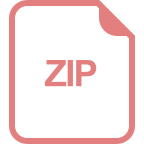
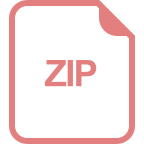
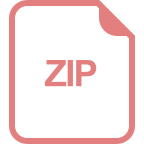
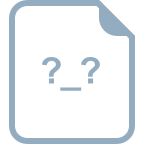
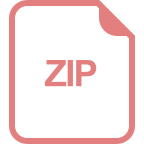
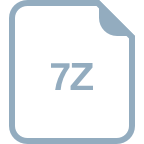
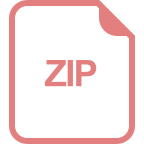
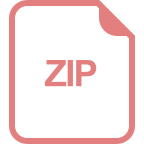
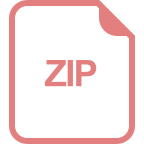