使用react和antd写一个表单
时间: 2024-05-08 19:15:31 浏览: 158
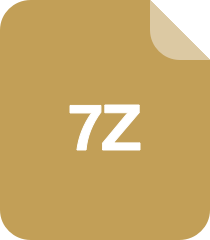
react+antd拖拽生成自定义表单.rar
以下是使用React和Antd编写表单的示例代码:
```
import React, { useState } from "react";
import { Form, Input, Button } from "antd";
const FormComponent = () => {
const [form] = Form.useForm();
const [loading, setLoading] = useState(false);
const onFinish = (values) => {
setLoading(true);
console.log("Received values of form: ", values);
// Send form data to backend
};
const onFinishFailed = (errorInfo) => {
console.log("Failed:", errorInfo);
};
return (
<Form
form={form}
name="basic"
initialValues={{ remember: true }}
onFinish={onFinish}
onFinishFailed={onFinishFailed}
>
<Form.Item
label="Username"
name="username"
rules={[
{ required: true, message: "Please input your username!" },
{ max: 15, message: "Username should be less than 15 characters!" },
]}
>
<Input />
</Form.Item>
<Form.Item
label="Password"
name="password"
rules={[
{ required: true, message: "Please input your password!" },
{ min: 6, message: "Password should be at least 6 characters!" },
]}
>
<Input.Password />
</Form.Item>
<Form.Item>
<Button type="primary" htmlType="submit" loading={loading}>
Submit
</Button>
</Form.Item>
</Form>
);
};
export default FormComponent;
```
在上面的代码中,我们使用了`Form`、`Input`和`Button`组件来构建表单。`Form`组件通过`useForm`钩子来获取`form`对象,`name`属性指定表单的名称,`initialValues`属性设置表单的初始值。`Form.Item`组件用于包装每个表单项,`label`属性设置表单项的标签,`name`属性设置表单项的名称,`rules`属性设置表单项的校验规则。`Input`组件用于输入文本,`Input.Password`组件用于输入密码。`Button`组件用于提交表单数据,`type`属性设置按钮的类型,`htmlType`属性设置按钮的提交类型,`loading`属性设置按钮是否处于加载状态。`onFinish`方法在表单提交成功后被调用,`onFinishFailed`方法在表单提交失败后被调用。我们可以在这两个方法中处理表单数据的提交和校验错误的处理。
阅读全文
相关推荐
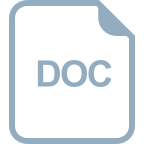
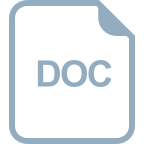


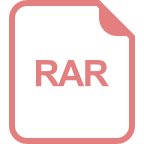
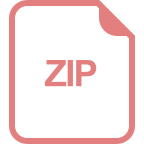
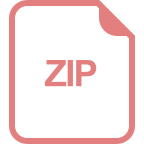
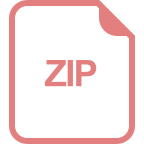
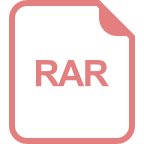
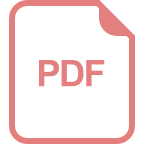
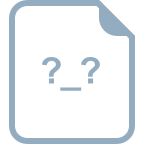
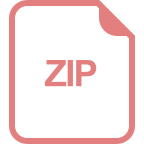





