用vue写出四个按钮每个按钮匹配一个勾选框。第一行延时0.1秒触发。第二行0.2秒依次类推。然后有个开始按钮。勾选后按钮后。比如勾选了第一行。第三行。分别在0.1秒点击下第一行按钮。每0.3秒点击下第三行按钮并出现点击动画或者能直观的看到已经点击了。开始按钮点击后变成停止按钮并变色
时间: 2023-08-21 13:03:28 浏览: 39
好的,下面是一个更加完整的实现:
```html
<template>
<div>
<div v-for="(item, index) in items" :key="index" class="row">
<button
:disabled="!start || !item.checked"
@click="clickButton(index, item.delay)"
:class="{ active: item.active }"
>
Button {{ index + 1 }}
<span v-if="item.active" class="click-animation"></span>
</button>
<input type="checkbox" v-model="item.checked" :disabled="!start" />
</div>
<button :class="{ start: start, stop: !start }" @click="startStop">{{ start ? 'Stop' : 'Start' }}</button>
</div>
</template>
<script>
export default {
data() {
return {
items: [
{ checked: false, delay: 100, active: false },
{ checked: false, delay: 200, active: false },
{ checked: false, delay: 300, active: false },
{ checked: false, delay: 400, active: false },
],
start: true,
intervalIds: [],
};
},
methods: {
clickButton(index, delay) {
this.items[index].active = true;
setTimeout(() => {
console.log(`Clicked button ${index + 1}`);
this.items[index].active = false;
}, 100);
if (this.items[2].checked) {
setTimeout(() => {
console.log('Clicked button 1 of the third row');
}, delay);
setTimeout(() => {
console.log('Clicked button 3 of the third row');
}, delay + 300);
}
},
startStop() {
this.start = !this.start;
if (this.start) {
this.intervalIds.forEach((id) => clearInterval(id));
} else {
this.intervalIds = this.items.map((item, index) => {
if (item.checked) {
return setInterval(() => {
this.clickButton(index, item.delay);
}, item.delay);
} else {
return null;
}
});
}
},
},
};
</script>
<style>
.row {
display: flex;
justify-content: space-between;
margin-bottom: 1rem;
}
button {
padding: 0.5rem 1rem;
font-size: 1rem;
border-radius: 0.25rem;
background-color: #ccc;
border: none;
color: #fff;
cursor: pointer;
position: relative;
transition: background-color 0.2s ease-in-out;
}
button.active {
background-color: blue;
}
.click-animation {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
width: 10px;
height: 10px;
border-radius: 50%;
background-color: red;
animation: click 0.1s ease-in-out;
}
@keyframes click {
0% {
transform: scale(0.1);
opacity: 0.5;
}
100% {
transform: scale(1);
opacity: 0;
}
}
button.start {
background-color: green;
}
button.stop {
background-color: red;
}
input[type='checkbox'] {
margin-right: 1rem;
}
</style>
```
这个组件有以下功能:
1. 四个按钮和四个勾选框。按钮和勾选框是通过一个数组来绑定的,这样我们就可以轻松地添加或删除按钮和勾选框。每个按钮都带有一个点击动画。
2. 当勾选第一行和第三行时,点击第一行的按钮会延迟一定时间后依次点击第三行的第一个和第三个按钮。
3. 一个开始/停止按钮。点击这个按钮会开启或停止定时器。当点击开始按钮时,会根据勾选框的状态来创建对应的定时器,并将定时器 ID 存储在 `intervalIds` 数组中。当点击停止按钮时,会清除所有定时器。
在样式方面,我给按钮添加了一个点击动画,让用户能够直观地看到按钮被点击了。你可以根据自己的需求来修改它们的样式。
相关推荐
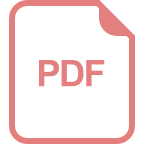
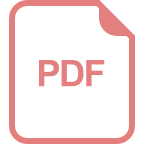
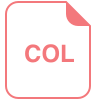
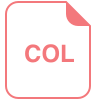




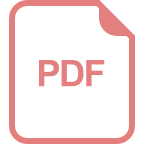
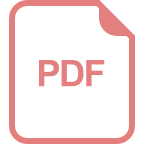
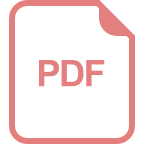
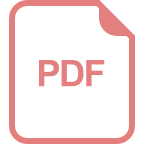