Python怎么将图片插入到word指定位置中
时间: 2024-05-02 16:19:42 浏览: 13
你可以使用 python-docx 模块来操作 Word 文档,使用 PIL(Python Imaging Library)库来处理图片。具体步骤如下:
1. 首先安装 python-docx 和 PIL
```
pip install python-docx
pip install Pillow
```
2. 打开 Word 文档并定位到需要插入图片的位置
```python
import docx
from docx.shared import Inches
# 打开 Word 文档
doc = docx.Document('example.docx')
# 定位到需要插入图片的位置
p = doc.add_paragraph()
r = p.add_run()
# 在定位位置插入图片
img_path = 'example.jpg'
pic = r.add_picture(img_path, width=Inches(3))
```
3. 保存 Word 文档
```python
doc.save('example.docx')
```
记得将 `img_path` 替换为你需要插入的图片路径,`width=Inches(3)` 表示图片宽度为 3 英寸。
相关问题
python怎么把数据写到word指定位置
你可以使用 python-docx 库来实现将数据写入到 Word 文档的指定位置。具体步骤如下:
1. 安装 python-docx 库:在命令行中输入 pip install python-docx。
2. 导入库:在 Python 脚本中导入 python-docx 库。
3. 打开 Word 文档:使用 Document() 函数打开要写入数据的 Word 文档。
4. 定位到指定位置:使用 paragraphs[index] 或 tables[index] 等方法定位到要写入数据的位置。
5. 写入数据:使用 add_run() 函数将数据写入到指定位置。
6. 保存文档:使用 save() 函数保存 Word 文档。
下面是一个示例代码:
```python
from docx import Document
# 打开 Word 文档
doc = Document('example.docx')
# 定位到指定位置
paragraph = doc.paragraphs[0]
# 写入数据
run = paragraph.add_run('Hello, World!')
# 保存文档
doc.save('example.docx')
```
这个示例代码将在 Word 文档的第一个段落中写入字符串 "Hello, World!"。你可以根据需要修改代码来实现将数据写入到其它位置。
python 批量将图片插入word
您可以使用Python中的docx库和Pillow库来实现此操作,以下是一些示例代码:
首先,您需要安装这两个库:
```
pip install python-docx
pip install Pillow
```
然后,您可以使用以下代码来将图像插入Word文档中:
```python
from docx import Document
from docx.shared import Inches
from PIL import Image
document = Document()
# 打开所有图片,并将它们插入到文档中
for i in range(1, 6):
image = Image.open(f'image_{i}.jpg')
width, height = image.size
document.add_picture(f'image_{i}.jpg', width=Inches(6), height=Inches(6*height/width))
# 保存文档
document.save('images.docx')
```
上面的代码打开名为`image_1.jpg`到`image_5.jpg`的五个图像,将其插入到Word文档中,并使用`Inches`指定图像的大小(保持宽高比)。最后,它将文档保存为`images.docx`文件。
希望这可以帮助您完成您的图像插入Word的任务!
相关推荐
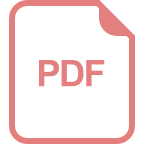












