How to connect to Http endpoint using Java programming
时间: 2023-10-09 15:05:23 浏览: 97
To connect to an HTTP endpoint using Java programming, you can use the HttpURLConnection class provided in the Java standard library. Here is an example code snippet:
```java
import java.net.HttpURLConnection;
import java.net.URL;
import java.io.BufferedReader;
import java.io.InputStreamReader;
public class HttpExample {
public static void main(String[] args) {
try {
// Create a URL object for the endpoint
URL url = new URL("http://example.com/api/endpoint");
// Create an HttpURLConnection object
HttpURLConnection con = (HttpURLConnection) url.openConnection();
// Set the request method (GET, POST, etc.)
con.setRequestMethod("GET");
// Read the response from the endpoint
BufferedReader in = new BufferedReader(new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
// Print the response to the console
System.out.println(response.toString());
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
In this example, we create a URL object for the endpoint we want to connect to, and then create an HttpURLConnection object with that URL. We set the request method to "GET", which is the most common method for retrieving data from an HTTP endpoint. We then read the response from the endpoint using a BufferedReader, and append each line of the response to a StringBuffer. Finally, we print the response to the console.
Note that this is just a basic example, and there are many different ways to connect to an HTTP endpoint in Java, depending on your specific requirements.
阅读全文
相关推荐
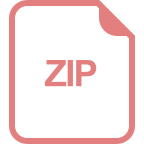
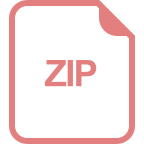
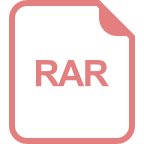
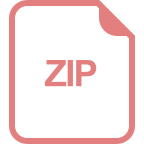
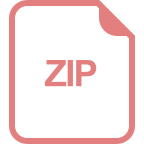
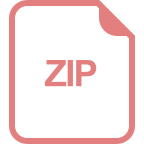
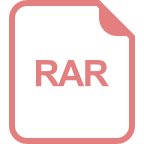
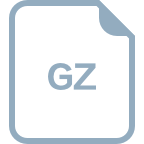
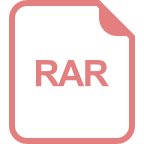