三、定义商品类Goods,包含单价 unitPrice和数量account两个属性,方法包括构造方法和价格计算方法totalPriceO。 定义接口VipPrice,包含 DISCOUNT属性和reducedPriceO方法,使VIP会 员享受商品价格8.5折待遇。 定义服装子类Clothing,它继承商品类Goods,并实现接口VipPrice,并有 服装样式style属性、构造方法和toString方法。 编写一个测试类,创建一种服装(180,2,“女装”),利用toString方法输出服装信息。 四、编写一个程序,从控制台读取用户的输入,并将其写入文本文件 input.txt中,直到用户输入"exit"为止。
时间: 2024-03-05 10:55:01 浏览: 26
好的,您的第一个问题是关于Java编程的。以下是回答:
1. 商品类Goods的定义如下:
```java
public class Goods {
private double unitPrice;
private int account;
public Goods(double unitPrice, int account) {
this.unitPrice = unitPrice;
this.account = account;
}
public double totalPrice() {
return unitPrice * account;
}
}
```
2. 接口VipPrice的定义如下:
```java
public interface VipPrice {
double DISCOUNT = 0.85;
double reducedPrice();
}
```
3. 服装子类Clothing的定义如下:
```java
public class Clothing extends Goods implements VipPrice {
private String style;
public Clothing(double unitPrice, int account, String style) {
super(unitPrice, account);
this.style = style;
}
@Override
public double reducedPrice() {
return totalPrice() * DISCOUNT;
}
@Override
public String toString() {
return "Clothing{" +
"unitPrice=" + unitPrice +
", account=" + account +
", style='" + style + '\'' +
", totalPrice=" + reducedPrice() +
'}';
}
}
```
4. 测试类的代码如下:
```java
import java.util.Scanner;
public class Test {
public static void main(String[] args) {
Clothing clothing = new Clothing(180, 2, "女装");
System.out.println(clothing.toString());
Scanner scanner = new Scanner(System.in);
try (PrintWriter writer = new PrintWriter(new FileWriter("input.txt"))) {
String input;
while (true) {
input = scanner.nextLine();
if (input.equals("exit")) {
break;
}
writer.println(input);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
在运行测试类时,将会输出服装信息:
```
Clothing{unitPrice=180.0, account=2, style='女装', totalPrice=306.0}
```
同时,测试类还会从控制台读取用户的输入,并将其写入文本文件 input.txt 中,直到用户输入"exit"为止。
相关推荐














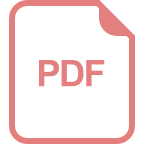