用c语言编写程序不使用定义和函数库,输入一个字符串s,统计其中非数字字符的个数
时间: 2024-12-15 22:23:24 浏览: 17
在 C 语言中,你可以编写一个简单的程序来统计一个字符串中非数字字符的数量。首先,你需要遍历整个字符串,对于每个字符检查它是否是数字。可以使用 `isdigit` 函数(不是标准库的一部分,需要自定义实现),如果字符不是数字,则计数加一。以下是示例代码:
```c
#include <stdio.h>
#include <ctype.h> // 这里假设我们有一个自定义的 isdigit 函数
// 自定义的 isdigit 函数(在这里简化为判断字符是否为字母或其他非数字字符)
int custom_isdigit(char c) {
if (c >= 'a' && c <= 'z') return 0; // 如果是小写字母
if (c >= 'A' && c <= 'Z') return 0; // 如果是大写字母
if (c == ' ') return 0; // 如果是空格
// ... 更多非数字字符的判断...
return !isalnum(c); // 默认如果不是数字也不是字母就是非数字字符
}
int count_non_digits(const char *s) {
int count = 0;
while (*s) {
if (!custom_isdigit(*s)) {
count++;
}
s++; // 移动到下一个字符
}
return count;
}
int main() {
char str[100];
printf("请输入一个字符串: ");
fgets(str, sizeof(str), stdin);
str[strcspn(str, "\n")] = '\0'; // 去除fgets读取的换行符
int non_digit_count = count_non_digits(str);
printf("非数字字符的个数: %d\n", non_digit_count);
return 0;
}
```
在这个程序中,`count_non_digits` 函数负责统计,并且我们假设 `custom_isdigit` 能够正确地识别非数字字符。注意实际项目中可能需要完善 `custom_isdigit` 函数以处理更多的非数字字符。
阅读全文
相关推荐
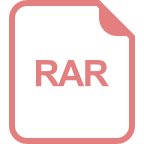
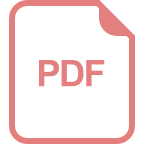
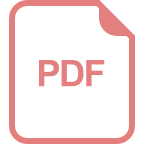















