1. 编写程序实现以下逻辑。 ① 抽象类Animal具有一个抽象方法walk。 ② 接口Flyable具有一个方法fly。 ③ 类Bird继承Animal并实现Flyable。 编写测试类BirdTest,在main方法中构造一个Bird对象并调用其walk和fly方法(重写这2个方法时,打印一行用于模拟的字符串即可)。 2. 接口MobilePhone定义了以下方法: void on(); // 开机 void off(); // 关机 void charge(); // 充电 分别采用如下两种方式创建两个实现MobilePhone接口的类的对象,并在测试类中分别对这两个对象调用上述方法。 ① 编写类IPhone实现MobilePhone接口。 ② 使用实现了MobilePhone接口的匿名内部类。 ③ 使用实现了MobilePhone接口的Lambda表达式。 3. 编写程序模拟用以表示直线的Line类,具体包含以下逻辑: ① 表示直线起点和终点的嵌套类Point,其包含描述横纵坐标的x、y字段。 ② 表示直线方向的嵌套枚举Direction,其包含描述若干方向的枚举常量。 在Line类中编写必要的代码,然后在测试类LineTest中构造几个Line对象并输出每个对象的有关信息。用Java代码写出上边的1、2、3
时间: 2024-03-06 21:51:04 浏览: 120
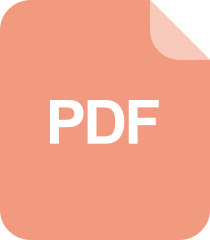
设计引导--一个鸭子游戏引发的设计理念(多态,继承,抽象,接口,策略者模式)
1.
```java
abstract class Animal {
public abstract void walk();
}
interface Flyable {
public void fly();
}
class Bird extends Animal implements Flyable {
@Override
public void walk() {
System.out.println("Bird is walking.");
}
@Override
public void fly() {
System.out.println("Bird is flying.");
}
}
public class BirdTest {
public static void main(String[] args) {
Bird bird = new Bird();
bird.walk();
bird.fly();
}
}
```
2.
```java
interface MobilePhone {
public void on();
public void off();
public void charge();
}
class IPhone implements MobilePhone {
@Override
public void on() {
System.out.println("IPhone is turned on.");
}
@Override
public void off() {
System.out.println("IPhone is turned off.");
}
@Override
public void charge() {
System.out.println("IPhone is charging.");
}
}
public class MobilePhoneTest {
public static void main(String[] args) {
MobilePhone phone1 = new IPhone();
phone1.on();
phone1.charge();
phone1.off();
MobilePhone phone2 = new MobilePhone() {
@Override
public void on() {
System.out.println("Anonymous phone is turned on.");
}
@Override
public void off() {
System.out.println("Anonymous phone is turned off.");
}
@Override
public void charge() {
System.out.println("Anonymous phone is charging.");
}
};
phone2.on();
phone2.charge();
phone2.off();
MobilePhone phone3 = () -> {
System.out.println("Lambda phone is turned on.");
System.out.println("Lambda phone is charging.");
System.out.println("Lambda phone is turned off.");
};
phone3.on();
phone3.charge();
phone3.off();
}
}
```
3.
```java
class Line {
static class Point {
int x;
int y;
public Point(int x, int y) {
this.x = x;
this.y = y;
}
}
enum Direction {
HORIZONTAL,
VERTICAL,
DIAGONAL
}
Point start;
Point end;
Direction direction;
public Line(Point start, Point end) {
this.start = start;
this.end = end;
if (start.x == end.x) {
direction = Direction.VERTICAL;
} else if (start.y == end.y) {
direction = Direction.HORIZONTAL;
} else {
direction = Direction.DIAGONAL;
}
}
@Override
public String toString() {
return "Line{" +
"start=" + start.x + "," + start.y +
", end=" + end.x + "," + end.y +
", direction=" + direction +
'}';
}
}
public class LineTest {
public static void main(String[] args) {
Line line1 = new Line(new Line.Point(0, 0), new Line.Point(0, 10));
Line line2 = new Line(new Line.Point(0, 0), new Line.Point(10, 0));
Line line3 = new Line(new Line.Point(0, 0), new Line.Point(10, 10));
System.out.println(line1);
System.out.println(line2);
System.out.println(line3);
}
}
```
阅读全文
相关推荐
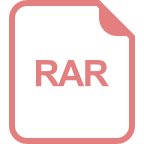
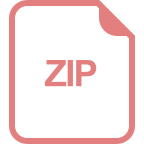




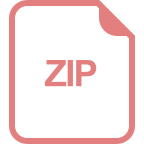
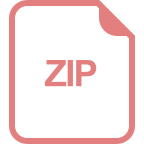
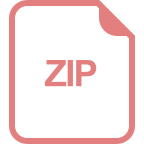
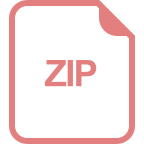
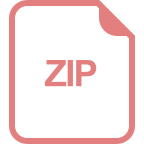
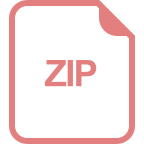
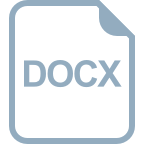