已知左相机拍摄图像上的两个二维坐标,请使用python-opencv,得到深度值
时间: 2023-12-03 20:44:50 浏览: 112
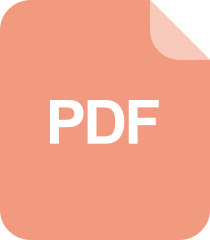
Python使用OpenCV进行标定
要得到深度值,需要知道两个二维坐标在三维空间中的坐标,然后计算它们之间的距离。这个过程需要使用相机的内参和外参,以及双目视差的计算方法。
以下是一个简单的示例代码,假设左相机内参矩阵为K1,畸变系数为D1,右相机内参矩阵为K2,畸变系数为D2,左相机到右相机的旋转矩阵为R,平移向量为T,左相机拍摄图像上的两个点的像素坐标为pt1和pt2:
```python
import cv2
import numpy as np
# 左相机内参矩阵和畸变系数
K1 = np.array([[focal_length, 0, cx], [0, focal_length, cy], [0, 0, 1]])
D1 = np.array([k1, k2, p1, p2, k3])
# 右相机内参矩阵和畸变系数
K2 = np.array([[focal_length, 0, cx], [0, focal_length, cy], [0, 0, 1]])
D2 = np.array([k1, k2, p1, p2, k3])
# 左相机到右相机的旋转矩阵和平移向量
R = np.array([[r11, r12, r13], [r21, r22, r23], [r31, r32, r33]])
T = np.array([t1, t2, t3])
# 左相机拍摄的图像和右相机拍摄的图像
img1 = cv2.imread('left.jpg')
img2 = cv2.imread('right.jpg')
# 将图像转换为灰度图
gray1 = cv2.cvtColor(img1, cv2.COLOR_BGR2GRAY)
gray2 = cv2.cvtColor(img2, cv2.COLOR_BGR2GRAY)
# 去畸变
undistort1 = cv2.undistort(gray1, K1, D1)
undistort2 = cv2.undistort(gray2, K2, D2)
# 计算双目视差
stereo = cv2.StereoBM_create(numDisparities=16, blockSize=15)
disparity = stereo.compute(undistort1, undistort2)
# 计算三维坐标
points1 = cv2.triangulatePoints(K1, np.hstack((np.eye(3), np.zeros((3, 1)))), pt1.reshape((2, 1)), K2, np.hstack((R, T)), pt2.reshape((2, 1)))
points1 /= points1[3]
points2 = cv2.triangulatePoints(K1, np.hstack((np.eye(3), np.zeros((3, 1)))), pt2.reshape((2, 1)), K2, np.hstack((R, T)), pt1.reshape((2, 1)))
points2 /= points2[3]
# 计算距离
distance = np.linalg.norm(points1[:3] - points2[:3])
print("距离为:", distance)
```
需要注意的是,这里使用的是简单的BM算法计算双目视差,实际上还有更加精确的算法,比如SGBM和深度学习方法。
阅读全文
相关推荐
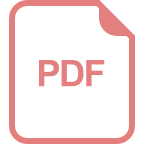
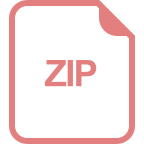















