springboot整合springsecurity+oauth2.0实现token认证
时间: 2023-07-26 15:04:13 浏览: 145
嗨!关于Spring Boot整合Spring Security和OAuth2.0实现token认证,你可以按照以下步骤进行操作:
1. 添加依赖:在你的Spring Boot项目的pom.xml文件中,添加Spring Security和OAuth2.0相关的依赖。
```xml
<dependencies>
<!-- Spring Security -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<!-- Spring Security OAuth2 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-oauth2-client</artifactId>
</dependency>
</dependencies>
```
2. 配置Spring Security:创建一个继承自WebSecurityConfigurerAdapter的配置类,并重写configure方法来配置Spring Security的行为。
```java
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/oauth2/**", "/login/**", "/logout/**")
.permitAll()
.anyRequest()
.authenticated()
.and()
.oauth2Login()
.loginPage("/login")
.and()
.logout()
.logoutSuccessUrl("/")
.invalidateHttpSession(true)
.clearAuthentication(true)
.deleteCookies("JSESSIONID");
}
}
```
在上述配置中,我们允许访问一些特定的URL(如/oauth2/**,/login/**和/logout/**),并保护所有其他URL。我们还设置了自定义的登录页面和注销成功后的跳转页面。
3. 配置OAuth2.0:创建一个继承自AuthorizationServerConfigurerAdapter的配置类,并重写configure方法来配置OAuth2.0的行为。
```java
@Configuration
@EnableAuthorizationServer
public class AuthorizationServerConfig extends AuthorizationServerConfigurerAdapter {
@Autowired
private AuthenticationManager authenticationManager;
@Override
public void configure(ClientDetailsServiceConfigurer clients) throws Exception {
clients
.inMemory()
.withClient("client_id")
.secret("client_secret")
.authorizedGrantTypes("authorization_code", "password", "refresh_token")
.scopes("read", "write")
.accessTokenValiditySeconds(3600)
.refreshTokenValiditySeconds(86400);
}
@Override
public void configure(AuthorizationServerEndpointsConfigurer endpoints) throws Exception {
endpoints
.authenticationManager(authenticationManager);
}
}
```
在上述配置中,我们使用内存存储客户端信息(client_id和client_secret),并配置了授权类型(如authorization_code、password和refresh_token)。我们还设置了访问令牌和刷新令牌的有效期。
4. 创建登录页面:创建一个HTML登录页面,用于用户进行身份验证并获取访问令牌。
```html
<!DOCTYPE html>
<html>
<head>
<title>Login</title>
</head>
<body>
<h2>Login</h2>
<form th:action="@{/login}" method="post">
<div>
<label for="username">Username:</label>
<input type="text" id="username" name="username" />
</div>
<div>
<label for="password">Password:</label>
<input type="password" id="password" name="password" />
</div>
<div>
<button type="submit">Login</button>
</div>
</form>
</body>
</html>
```
5. 处理登录请求:创建一个控制器来处理登录请求,并在登录成功后重定向到受保护的资源。
```java
@Controller
public class LoginController {
@GetMapping("/login")
public String showLoginForm() {
return "login";
}
@PostMapping("/login")
public String loginSuccess() {
return "redirect:/protected-resource";
}
}
```
在上述控制器中,我们使用@GetMapping注解来处理GET请求,@PostMapping注解来处理POST请求。登录成功后,我们将用户重定向到受保护的资源。
这样,你就完成了Spring Boot整合Spring Security和OAuth2.0实现token认证的配置。你可以根据自己的需求进行进一步的定制和扩展。希望对你有所帮助!如果你有任何疑问,请随时问我。
阅读全文
相关推荐
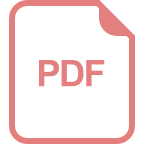
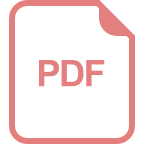
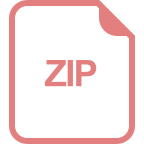
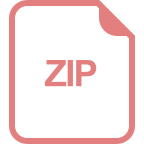
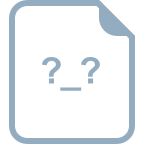
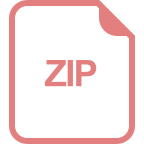


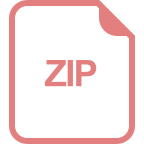
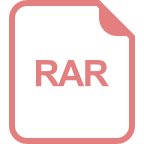
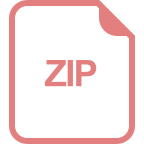
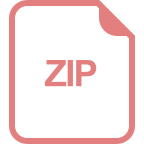
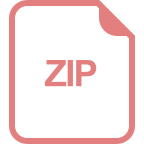
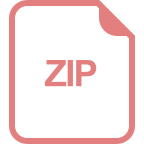
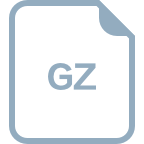

