vue3+ts+element实现导入Excel
时间: 2023-08-23 09:28:51 浏览: 207
在Vue 3中使用TypeScript和ElementUI实现导入Excel需要安装xlsx、@types/xlsx和element-ui两个包,可以使用以下命令进行安装:
```
npm install xlsx @types/xlsx element-ui --save
```
在Vue组件中,可以使用以下代码来读取Excel文件:
```typescript
<template>
<el-upload
class="upload-excel"
:show-file-list="false"
:on-change="onFileChange"
:before-upload="beforeUpload"
>
<el-button>
<i class="el-icon-upload"></i>
选择文件
</el-button>
</el-upload>
</template>
<script lang="ts">
import { defineComponent } from 'vue';
import * as XLSX from 'xlsx';
export default defineComponent({
data() {
return {
excelData: []
}
},
methods: {
beforeUpload(file: any) {
const fileType = file.type;
const validTypes = ['application/vnd.ms-excel', 'application/vnd.openxmlformats-officedocument.spreadsheetml.sheet'];
if (validTypes.indexOf(fileType) === -1) {
this.$message.error('只支持Excel文件');
return false;
}
return true;
},
onFileChange(event: any) {
const file = event.file.raw;
const reader = new FileReader();
reader.onload = (event: any) => {
const data = new Uint8Array(event.target.result);
const workbook = XLSX.read(data, { type: 'array' });
const sheetName = workbook.SheetNames[0];
const sheet = workbook.Sheets[sheetName];
const excelData = XLSX.utils.sheet_to_json(sheet, { header: 1 });
this.excelData = excelData;
this.$message.success('文件上传成功');
};
reader.readAsArrayBuffer(file);
}
}
});
</script>
```
在模板中,使用ElementUI的`<el-upload>`组件来实现文件上传。在`beforeUpload`方法中判断文件类型,只允许上传Excel文件。在`onFileChange`方法中读取Excel文件,并将数据存储在`excelData`中,并使用`this.$message.success`来提示上传成功。
注意:在使用TypeScript的时候,需要对组件中的方法、参数、返回值进行类型定义。
阅读全文
相关推荐
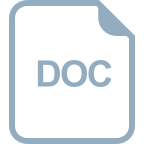
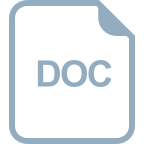
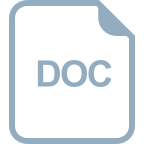
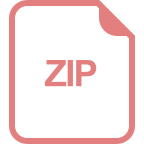
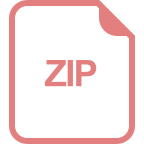
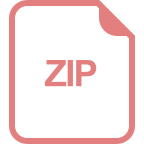
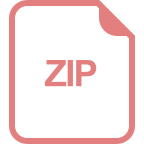
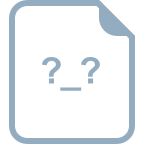
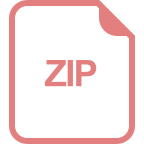
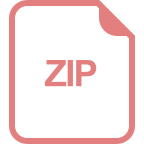
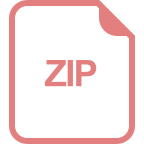
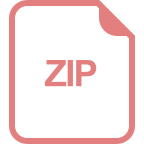
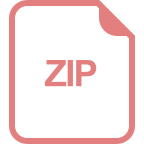
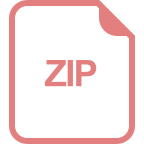
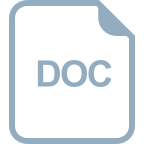
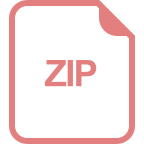
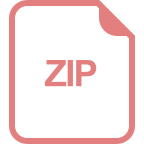
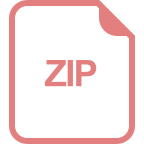
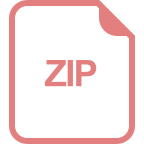