写一下工件gabor特征提取的Python代码
时间: 2023-11-21 15:11:01 浏览: 144
以下是用Python实现的工件Gabor特征提取代码:
```
import numpy as np
import cv2
def get_gabor_kernel(ksize, theta, sigma, lambd, gamma):
"""
function to generate Gabor kernel
"""
sigma_x = sigma
sigma_y = float(sigma) / gamma
# Bounding box
nstds = 3 # Number of standard deviation sigma
xmax = max(abs(nstds * sigma_x * np.cos(theta)), abs(nstds * sigma_y * np.sin(theta)))
xmax = np.ceil(max(1, xmax))
ymax = max(abs(nstds * sigma_x * np.sin(theta)), abs(nstds * sigma_y * np.cos(theta)))
ymax = np.ceil(max(1, ymax))
xmin = -xmax
ymin = -ymax
(y, x) = np.meshgrid(np.arange(ymin, ymax + 1), np.arange(xmin, xmax + 1))
# Rotation
x_theta = x * np.cos(theta) + y * np.sin(theta)
y_theta = - x * np.sin(theta) + y * np.cos(theta)
# Gaussian component
gb = np.exp(-0.5 * (x_theta ** 2 / sigma_x ** 2 + y_theta ** 2 / sigma_y ** 2))
# Sinusoidal component
gb *= np.cos(2 * np.pi / lambd * x_theta)
return gb
def get_gabor_features(img, ksize, thetas, sigmas, lambds, gammas):
"""
function to extract Gabor features from an image
"""
features = []
gray_img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
for theta in thetas:
for sigma in sigmas:
for lambd in lambds:
for gamma in gammas:
kernel = get_gabor_kernel(ksize, theta, sigma, lambd, gamma)
filtered = cv2.filter2D(gray_img, cv2.CV_8UC3, kernel)
features.append(filtered)
return features
```
其中,`ksize`是Gabor核的大小,`thetas`是角度值,`sigmas`是Gabor核的尺度,`lambds`是Gabor函数的波长,`gammas`是Gabor核的椭圆率系数(aspect ratio)。这段代码会对输入图像进行Gabor滤波,并返回滤波后的特征。
阅读全文
相关推荐




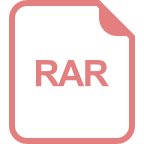
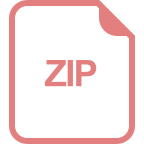
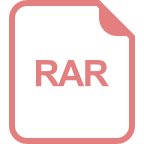
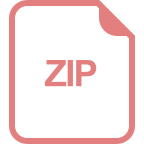
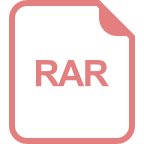
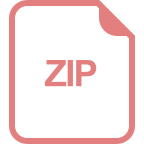
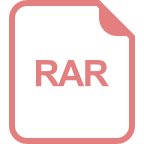



