simclr的代码实现
时间: 2023-09-02 16:11:41 浏览: 115
SimCLR是一种自监督学习方法,它使用了对比学习的思想。以下是SimCLR的代码实现:
1. 导入必要的库和模块
```python
import tensorflow as tf
from tensorflow.keras import layers
from tensorflow.keras import Model
from tensorflow.keras.optimizers import Adam
from tensorflow.keras.callbacks import ModelCheckpoint
```
2. 定义模型
```python
# 定义模型
def simclr_model(input_shape, num_classes):
# ResNet50 骨干网络
base_model = tf.keras.applications.ResNet50(input_shape=input_shape, include_top=False, weights=None)
# 去掉头部
x = layers.GlobalAveragePooling2D()(base_model.output)
# 添加两个全连接层
x = layers.Dense(512, activation='relu')(x)
x = layers.Dense(128, activation='relu')(x)
# 归一化处理,得到特征向量
x = layers.Normalization()(x)
# 输出特征向量
outputs = layers.Dense(num_classes)(x)
# 构建模型
model = Model(inputs=base_model.input, outputs=outputs)
return model
```
3. 定义对比学习损失函数
```python
# 对比学习损失函数
def contrastive_loss(y_true, y_pred):
# y_true 是一个全是1的向量
# y_pred 是两个特征向量的点积
# 对角线上的点积被忽略
temperature = 0.5
batch_size = tf.shape(y_pred)[0]
# 计算相似度矩阵
similarity_matrix = tf.matmul(y_pred, y_pred, transpose_b=True)
# 对角线上的相似度被忽略
mask = tf.eye(batch_size, dtype=tf.float32)
similarity_matrix = tf.multiply(similarity_matrix, 1 - mask)
# 计算正样本
positives = tf.linalg.diag_part(similarity_matrix)
# 计算负样本
negatives = tf.math.log(tf.reduce_sum(tf.exp(similarity_matrix / temperature), axis=1)) + tf.math.log(1 - tf.exp(positives / temperature))
# 计算损失
loss = tf.reduce_mean(positives / temperature - negatives / temperature)
return loss
```
4. 定义数据处理函数
```python
# 数据处理函数
def preprocess_image(image):
image = tf.image.resize(image, (224, 224))
image = tf.image.random_flip_left_right(image)
image = tf.image.random_brightness(image, max_delta=0.5)
image = tf.keras.applications.resnet50.preprocess_input(image)
return image
# 数据集处理函数
def prepare_dataset(ds, shuffle=False, augment=False, batch_size=32):
# 数据集预处理
ds = ds.map(lambda x: (preprocess_image(x[0]), preprocess_image(x[1])), num_parallel_calls=tf.data.AUTOTUNE)
# 数据打乱
if shuffle:
ds = ds.shuffle(buffer_size=10000)
# 数据增强
if augment:
ds = ds.map(lambda x, y: (tf.image.random_flip_left_right(x), tf.image.random_flip_left_right(y)), num_parallel_calls=tf.data.AUTOTUNE)
# 数据批处理
ds = ds.batch(batch_size)
# 数据预取
ds = ds.prefetch(tf.data.AUTOTUNE)
return ds
```
5. 定义训练函数
```python
# 训练函数
def train_simclr(train_ds, val_ds, epochs=10, batch_size=32):
# 定义模型
model = simclr_model((224, 224, 3), 128)
# 编译模型
model.compile(optimizer=Adam(learning_rate=0.001), loss=contrastive_loss)
# 定义回调函数
callbacks = [ModelCheckpoint('simclr_checkpoint.h5', save_best_only=True)]
# 训练模型
model.fit(prepare_dataset(train_ds, shuffle=True, augment=True, batch_size=batch_size),
epochs=epochs,
validation_data=prepare_dataset(val_ds, shuffle=False, augment=False, batch_size=batch_size),
callbacks=callbacks)
return model
```
6. 加载数据集并训练模型
```python
# 加载数据集
train_ds = tf.data.Dataset.from_tensor_slices((train_images, train_images))
val_ds = tf.data.Dataset.from_tensor_slices((val_images, val_images))
# 训练模型
model = train_simclr(train_ds, val_ds)
```
以上就是SimCLR的代码实现。
相关推荐
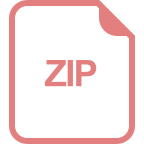
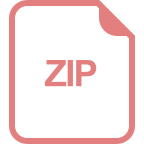














