C编程实现<key value>存储程序模块,向外提供创建和增删改查接口功
时间: 2024-10-13 21:10:00 浏览: 32
在C语言中,实现一个简单的键值对存储程序模块通常可以使用结构体(struct)和动态内存管理,例如使用`malloc`和`free`函数。这里有一个基础的例子,我们创建一个名为`KeyValueStore`的模块:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义一个键值对结构体
typedef struct {
char* key; // 键
char* value; // 值
} KeyValue;
// 创建一个新的键值对
KeyValue* create_pair(char* key, char* value) {
KeyValue* pair = (KeyValue*) malloc(sizeof(KeyValue));
if (pair != NULL) {
pair->key = strdup(key);
pair->value = strdup(value);
}
return pair;
}
// 添加键值对到存储中
void add_to_store(KeyValue** store, KeyValue* pair) {
*store = realloc(*store, sizeof(KeyValue)*(sizeof(*store)+1));
(*store)[sizeof(*store)-1] = *pair;
}
// 删除指定键的键值对
void delete_from_store(KeyValue** store, const char* key) {
int i;
for (i = 0; i < sizeof(*store); i++) {
if (strcmp((*store)[i].key, key) == 0) {
free((*store)[i].key);
free((*store)[i].value);
memmove(&(*store)[i], &(*store)[i+1], (sizeof(*store) - i - 1) * sizeof(KeyValue));
break;
}
}
if (i == sizeof(*store)) {
printf("Key not found.\n");
}
*store = realloc(*store, sizeof(KeyValue)*i);
}
// 查找并打印键对应的值
void lookup_in_store(const KeyValue* store, const char* key) {
int i;
for (i = 0; i < sizeof(store); i++) {
if (strcmp(store[i].key, key) == 0) {
printf("Key: %s, Value: %s\n", key, store[i].value);
return;
}
}
printf("Key not found.\n");
}
int main() {
// 初始化空存储数组
KeyValue* store = NULL;
// 使用示例
KeyValue* pair1 = create_pair("name", "John");
add_to_store(&store, pair1);
delete_from_store(&store, "name"); // 删除键为"name"的项
lookup_in_store(store, "unknown"); // 查找不存在的键
return 0;
}
```
这个例子中,我们创建了`create_pair`, `add_to_store`, `delete_from_store`和`lookup_in_store`函数来操作`KeyValueStore`。注意这是个简化的版本,实际应用中可能会需要考虑错误处理、数据持久化等细节。
阅读全文
相关推荐
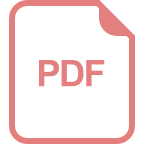
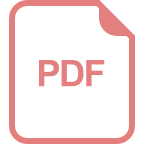
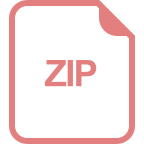

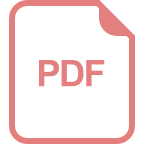
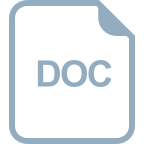
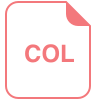
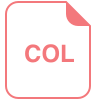
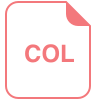
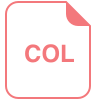
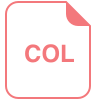
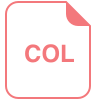






