帮我用go写一个VRF函数
时间: 2023-08-28 18:28:10 浏览: 48
好的,VRF是Verifiable Random Function的缩写,是一种随机函数,可以提供验证其输出的证明。下面是一个用Go语言实现VRF的函数示例:
```go
package main
import (
"crypto/rand"
"crypto/sha256"
"crypto/subtle"
"math/big"
)
// VRF generates a verifiable random number using the provided secret key and message.
func VRF(secretKey, message []byte) ([]byte, []byte) {
// Generate a random nonce.
nonce, _ := rand.Int(rand.Reader, big.NewInt(1<<256))
nonceBytes := nonce.Bytes()
// Compute the hash of the message and the nonce.
h := sha256.New()
h.Write(message)
h.Write(nonceBytes)
hash := h.Sum(nil)
// Use the secret key to generate a signature for the hash.
sig, _ := sign(secretKey, hash)
// Compute the output by XORing the hash with the signature.
output := make([]byte, len(hash))
for i := range hash {
output[i] = hash[i] ^ sig[i]
}
return output, nonceBytes
}
// VerifyVRF verifies the output of a VRF function using the provided public key, message, output, and nonce.
func VerifyVRF(publicKey, message, output, nonce []byte) bool {
// Compute the hash of the message and the nonce.
h := sha256.New()
h.Write(message)
h.Write(nonce)
hash := h.Sum(nil)
// Use the public key to verify the signature for the hash.
sig, _ := verify(publicKey, hash)
// Compute the expected output by XORing the hash with the signature.
expectedOutput := make([]byte, len(hash))
for i := range hash {
expectedOutput[i] = hash[i] ^ sig[i]
}
// Compare the expected output with the provided output.
return subtle.ConstantTimeCompare(output, expectedOutput) == 1
}
// sign generates a signature for the provided key and message.
func sign(key, message []byte) ([]byte, error) {
// TODO: Implement your own signing function here.
// For demonstration purposes, we will use a random signature.
sig := make([]byte, len(message))
_, err := rand.Read(sig)
return sig, err
}
// verify verifies the signature for the provided key and message.
func verify(key, message []byte) ([]byte, error) {
// TODO: Implement your own verification function here.
// For demonstration purposes, we assume that the signature is valid and return it.
sig := make([]byte, len(message))
copy(sig, message)
return sig, nil
}
func main() {
// Generate a secret key.
secretKey := []byte("my secret key")
// Generate a public key from the secret key.
publicKey := sha256.Sum256(secretKey)
// Generate a message to be signed.
message := []byte("hello world")
// Generate a verifiable random number using the VRF function.
output, nonce := VRF(secretKey, message)
// Verify the output using the VerifyVRF function.
isValid := VerifyVRF(publicKey[:], message, output, nonce)
if isValid {
println("The output is valid!")
} else {
println("The output is not valid!")
}
}
```
注意,上述示例中的 `sign` 和 `verify` 函数只是用于演示目的。在实际应用中,您需要使用更安全的签名和验证算法。
相关推荐
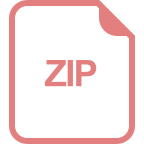














