C#读取psd文件整体图像尺寸
时间: 2023-07-12 07:22:00 浏览: 239
要读取 PSD 文件的整体图像尺寸,可以使用 C# 中的 PhotoshopFile 类库。这个类库是由 CodeTitans 开发的,可以方便地读取 PSD 文件的元数据和图像数据。
以下是读取 PSD 文件整体图像尺寸的代码示例:
```csharp
using CodeTitans.JSon;
// 读取 PSD 文件
var psdFile = new PhotoshopFile(@"C:\path\to\file.psd");
// 获取整体图像尺寸
var docWidth = psdFile.DocumentSize.Width;
var docHeight = psdFile.DocumentSize.Height;
// 输出整体图像尺寸
Console.WriteLine($"Document Size: {docWidth} x {docHeight}");
```
在这个示例中,我们首先创建了一个 `PhotoshopFile` 对象来读取 PSD 文件。然后,我们可以使用 `DocumentSize` 属性来获取整体图像的尺寸。最后,我们将整体图像的尺寸输出到控制台中。
需要注意的是,使用 `PhotoshopFile` 类库需要先安装 CodeTitans.JSon 包,可以通过 NuGet 包管理器来安装。同时,需要引入 `CodeTitans.PCS` 和 `CodeTitans.JSon` 命名空间。
相关问题
C#不使用Photoshop SDK读取PSD长宽
如果你不想使用Photoshop SDK,也可以使用C#内置的System.Drawing.Imaging命名空间来读取PSD文件的长宽。下面是一个示例代码,它演示了如何打开一个PSD文件,读取其长宽,并关闭文件:
```csharp
using System.Drawing;
using System.Drawing.Imaging;
class Program
{
static void Main(string[] args)
{
// Replace "path/to/file.psd" with the actual path to your PSD file.
using (Image image = Image.FromFile("path/to/file.psd"))
{
int width = image.Width;
int height = image.Height;
Console.WriteLine("Width: {0}, Height: {1}", width, height);
}
}
}
```
请注意,在使用这个示例代码之前,你需要添加对System.Drawing.dll程序集的引用。另外,System.Drawing.Imaging命名空间也提供了许多其他有用的类和方法,用于处理图像文件,例如获取像素值、缩放图像等等。
C#不使用Photoshop导出PSD图层
如果你不想使用Photoshop SDK来导出PSD图层,你可以使用第三方的C#库来实现。比如使用NReco.ImageSharp库,这是一个开源的图像处理库,支持读取和写入PSD文件。
以下是一些参考步骤:
1. 安装NReco.ImageSharp库,在Visual Studio中打开NuGet包管理器,搜索NReco.ImageSharp并安装。
2. 加载PSD图像文件,你可以使用`Image.Load()`方法来加载PSD文件。例如:
```
string filePath = "your_path_to_psd_file";
using (var img = Image.Load(filePath)) {
// 处理图像
}
```
3. 导出图层,使用`Image.Save()`方法来保存图层。例如:
```
string layerPath = "your_path_to_export_layer";
using (var layer = img.Frames[0].Clone()) {
layer.Save(layerPath);
}
```
需要注意的是,NReco.ImageSharp库虽然支持读写PSD文件,但是它并不支持所有的PSD文件格式和特性,因此在使用时需要注意文件格式和兼容性问题。
阅读全文
相关推荐
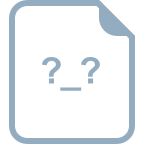
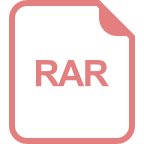
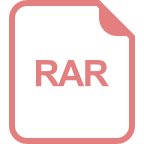
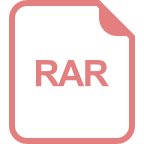
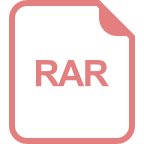
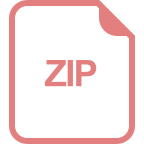
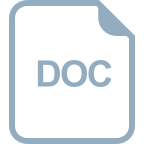
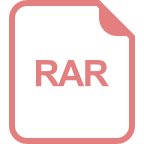
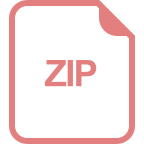
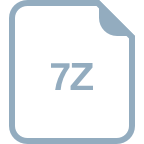
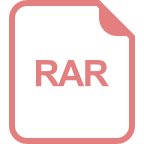
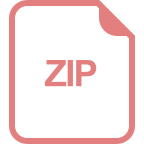
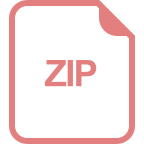
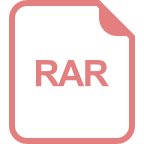
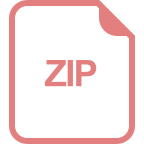