利用python写一个简易的图书管理系统,包括查询图书,借阅图书,归还图书,退出系统
时间: 2024-03-18 14:42:13 浏览: 61
好的,我可以帮您实现这个简易的图书管理系统。首先,我们需要建立一个包含图书信息的列表,包括图书名称、作者、出版社和是否已被借阅。
接下来是代码实现:
```python
# 图书信息列表
books = [
{'name': 'Python基础教程', 'author': 'Magnus Lie Hetland', 'publisher': '人民邮电出版社', 'borrowed': False},
{'name': 'Python编程快速上手', 'author': 'Eric Matthes', 'publisher': '人民邮电出版社', 'borrowed': False},
{'name': 'Python数据分析实战', 'author': '徐靖峰', 'publisher': '人民邮电出版社', 'borrowed': False},
{'name': 'Python网络爬虫', 'author': '崔庆才', 'publisher': '人民邮电出版社', 'borrowed': False},
{'name': 'Python爬虫开发与项目实战', 'author': '陈宇翔', 'publisher': '人民邮电出版社', 'borrowed': False}
]
# 查询图书
def search_book():
while True:
keyword = input("请输入要查询的关键词:")
results = []
for book in books:
if keyword in book['name'] or keyword in book['author'] or keyword in book['publisher']:
results.append(book)
if results:
print("查询结果如下:")
for result in results:
print(result)
break
else:
print("没有找到符合条件的图书,请重新输入关键词!")
# 借阅图书
def borrow_book():
while True:
book_name = input("请输入要借阅的图书名称:")
for book in books:
if book['name'] == book_name and not book['borrowed']:
book['borrowed'] = True
print("借阅成功!")
return
print("该图书已被借阅或不存在,请重新输入!")
# 归还图书
def return_book():
while True:
book_name = input("请输入要归还的图书名称:")
for book in books:
if book['name'] == book_name and book['borrowed']:
book['borrowed'] = False
print("归还成功!")
return
print("该图书未被借阅或不存在,请重新输入!")
# 退出系统
def exit_system():
print("已退出系统!")
# 主程序
def main():
while True:
print("请选择操作:")
print("1. 查询图书")
print("2. 借阅图书")
print("3. 归还图书")
print("4. 退出系统")
choice = input()
if choice == '1':
search_book()
elif choice == '2':
borrow_book()
elif choice == '3':
return_book()
elif choice == '4':
exit_system()
break
else:
print("无效的选择,请重新输入!")
if __name__ == "__main__":
main()
```
以上代码实现了简易的图书管理系统,包括查询图书、借阅图书、归还图书和退出系统等功能。需要注意的是,此处仅作演示之用,实际应用中应该使用数据库等方式存储图书信息,以便于管理和查询。
阅读全文
相关推荐
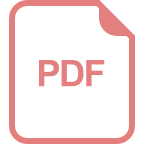
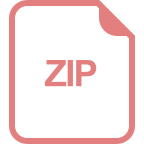





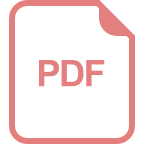
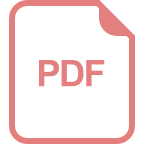
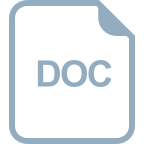



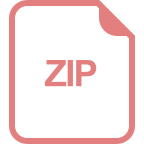
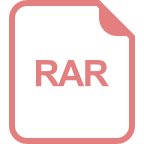
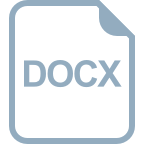