if params.grad is not None: params.grad.zero_()
时间: 2024-05-21 12:10:15 浏览: 175
This line of code checks if the gradient of the parameters (params) is not None. If it is not None, it sets the gradient to zero using the zero_() method. This is a common step in training neural networks using backpropagation, where the gradients are accumulated during each forward pass and used to update the weights during the backward pass. Setting the gradient to zero before the next forward pass ensures that the gradients are not accumulated across multiple batches or iterations.
相关问题
if params.grad is not None: params.grad.zero_()是什么意思
这段代码是用于清空梯度值的。当计算图中的梯度值已经被累加过一次后,需要在下一次计算梯度之前将其清零。这样做可以防止梯度值的叠加影响下一次的计算结果。
具体来说,如果params.grad不为None,即params存在梯度值,那么就使用params.grad.zero_()将其清零。其中,params.grad是一个张量,代表params的梯度值,zero_()是一个方法,用于将张量的所有元素设置为0。
现在我们有一个要优化的网络,让我们看看PyTorch的优化器类。为了实现一个优化器,我们需要实现step()函数来实际更新参数。帮我按照上面的要求补充下面的代码 class GD(Optimizer): def __init__(self, params, lr=0.2) -> None: super().__init__(params, {'lr': lr}) self.lr = lr def step(self): # do not consider the next steps for gradient calculations with torch.no_grad(): # iter over all parameters for p in self.param_groups[0]['params']: # if the gradient is set, update it if p.grad is not None: # update parameters # hint: in torch each function (e.g. Tensor.add()) has an inplace variant # which modifies the tensor inplace: Tensor.add_() ...
好的,请参考下面的完整代码:
```python
class GD(Optimizer):
def __init__(self, params, lr=0.2) -> None:
super().__init__(params, {'lr': lr})
self.lr = lr
def step(self):
# do not consider the next steps for gradient calculations
with torch.no_grad():
# iter over all parameters
for p in self.param_groups[0]['params']:
# if the gradient is set, update it
if p.grad is not None:
# update parameters
# hint: in torch each function (e.g. Tensor.add()) has an inplace variant
# which modifies the tensor inplace: Tensor.add_()
p.add_(self.lr * (-p.grad))
# clear gradients
p.grad.zero_()
```
这段代码中,我们定义了一个名为`GD`的类来实现梯度下降优化器。在构造函数中,我们首先调用`super()`函数来初始化父类`Optimizer`。然后我们定义了学习率`lr`,并将其保存在对象属性中。
在`step()`函数中,我们首先使用`with torch.no_grad():`语句块来告诉PyTorch在下面的计算中不需要计算梯度。然后我们使用`self.param_groups[0]['params']`来获取网络中所有的参数,并使用循环逐个更新它们。在更新参数时,我们使用`p.add_(self.lr * (-p.grad))`来使用梯度下降更新参数。最后,我们使用`p.grad.zero_()`来清除计算的梯度,以便下一次计算。
阅读全文
相关推荐
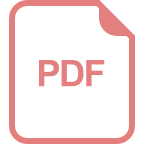
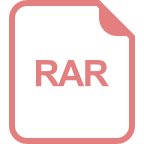
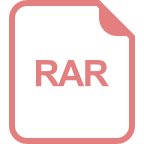













