python ip = findall("(?<=\()[\d|/.]+(?=\))", log) 是什么意思
时间: 2023-03-29 16:04:02 浏览: 102
这个 Python 代码的作用是从一个日志中找到括号内的 IP 地址。具体来说,它使用正则表达式中的 lookbehind 和 lookahead,匹配括号内的数字、斜杠和小数点,然后返回匹配到的 IP 地址。
相关问题
python200行代码爬虫
### Python 爬虫示例约200行代码
下面展示了一个较为完整的Python爬虫程序,该程序不仅能够抓取网页内容,还能处理更复杂的逻辑,比如登录验证、数据存储以及异常处理等。此例子综合运用了`requests`库用于网络请求,`BeautifulSoup`库解析HTML文档,并引入了一些额外的功能模块以增强实用性。
```python
import requests
from bs4 import BeautifulSoup
import os
import time
import json
class WebCrawler:
def __init__(self, base_url='https://example.com'):
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64)',
'Accept-Language': 'en-US,en;q=0.9'
}
self.session = requests.Session()
self.base_url = base_url
self.headers = headers
def login(self, username, password):
"""模拟登录"""
url = f"{self.base_url}/login"
payload = {'username': username, 'password': password}
try:
resp = self.session.post(url, data=payload, headers=self.headers)
if resp.status_code == 200 and "success" in resp.text.lower():
print('Login successful.')
return True
else:
print(f'Failed to log in with status code {resp.status_code}.')
return False
except Exception as e:
print(f"An error occurred during login: {e}")
return None
def fetch_page_content(self, page_number=1):
"""获取指定页数的内容"""
target_url = f'{self.base_url}?page={page_number}'
try:
response = self.session.get(target_url, headers=self.headers)
soup = BeautifulSoup(response.content, 'html.parser')
articles = []
for article_div in soup.find_all('div', class_='article'):
title = article_div.h2.a.string.strip() or ''
link = article_div.h2.a['href'] or '#'
summary_p = article_div.p
summary_text = summary_p.string.strip() if summary_p else ''
articles.append({
'title': title,
'link': link,
'summary': summary_text
})
return articles
except Exception as ex:
print(f"Error fetching content from page {page_number}: ", ex)
return []
def save_data_to_file(self, filename="data.json", pages_range=(1, 3)):
"""保存多页的数据到本地JSON文件中"""
all_articles = []
start_time = time.time()
for i in range(pages_range[0], pages_range[-1]+1):
current_articles = crawler.fetch_page_content(i)
# 防止过快访问服务器引起封禁IP的风险
sleep_duration = min((i - pages_range[0]) * 0.5, 2)
time.sleep(sleep_duration)
all_articles.extend(current_articles)
end_time = time.time()
elapsed_time = round(end_time - start_time, 2)
with open(filename, mode='w+', encoding='utf8') as file_obj:
json.dump(all_articles, file_obj, ensure_ascii=False, indent=4)
print(f'Successfully saved {len(all_articles)} records into "{filename}" within {elapsed_time} seconds.')
if __name__ == '__main__':
USERNAME = '' # 用户名
PASSWORD = '' # 密码
crawler = WebCrawler(base_url='https://targetwebsite.example.org')
logged_in = crawler.login(username=USERNAME, password=PASSWORD)
if not logged_in:
exit(-1)
crawler.save_data_to_file(filename='./output/articles_info.json', pages_range=(1, 5))
```
这段代码实现了如下功能:
- 创建了一个名为 `WebCrawler` 的类来封装主要操作方法。
- 使用会话(`Session`)保持连接状态以便于后续可能涉及到的身份认证过程。
- 实现了基本的错误捕捉机制,在遇到问题时可以给出提示而不是直接崩溃。
- 添加了合理的延时策略,防止因频繁请求而被目标站点屏蔽 IP 地址。
- 将收集到的文章信息存入 JSON 文件内,方便日后分析或再利用这些资料[^1]。
python爬虫的基本约定
Python爬虫的基本约定主要包括以下几个方面:
1. **尊重robots.txt**:在爬取网站之前,首先要检查网站的robots.txt文件。这个文件规定了哪些页面允许爬虫访问,哪些不允许。遵守robots.txt是爬虫的基本道德规范。
2. **控制爬取速度**:为了避免对目标网站造成过大压力,爬虫应该控制爬取速度。可以通过设置请求间隔时间来实现这一点。
3. **设置合适的请求头**:为了模拟浏览器行为,爬虫应该设置合适的请求头,如User-Agent、Referer等。这样可以减少被网站屏蔽的风险。
4. **处理异常和错误**:爬虫在运行过程中可能会遇到各种异常和错误,如网络连接超时、页面解析错误等。应该对这些异常进行处理,确保爬虫的稳定性。
5. **数据存储**:爬取到的数据需要存储起来,常见的方式有保存为CSV文件、存储到数据库中等。根据需求选择合适的数据存储方式。
6. **合法合规**:爬虫的使用必须遵守相关法律法规,不能用于非法用途,如侵犯他人隐私、盗取数据等。
7. **使用代理**:为了避免IP被封禁,可以使用代理IP。代理IP可以隐藏真实IP地址,减少被封禁的风险。
8. **日志记录**:记录爬虫的运行日志,包括爬取的时间、URL、状态等信息。日志记录有助于调试和维护爬虫。
以下是一个简单的Python爬虫示例,展示了上述基本约定的实现:
```python
import requests
from bs4 import BeautifulSoup
import time
import logging
# 设置日志
logging.basicConfig(filename='spider.log', level=logging.INFO)
# 设置请求头
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
# 爬取函数
def crawl(url):
try:
response = requests.get(url, headers=headers, timeout=10)
response.raise_for_status()
response.encoding = response.apparent_encoding
return response.text
except requests.RequestException as e:
logging.error(f"Request failed: {e}")
return None
# 解析函数
def parse(html):
soup = BeautifulSoup(html, 'html.parser')
titles = soup.find_all('title')
for title in titles:
print(title.get_text())
# 主函数
def main():
url = 'http://example.com'
html = crawl(url)
if html:
parse(html)
time.sleep(1) # 控制爬取速度
if __name__ == '__main__':
main()
```
阅读全文
相关推荐








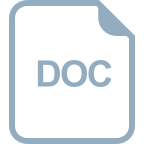






