用python实现基于概率分布的超声图像与自然图像性质差异分析,不用greycomatrix和greycoprops,使用opencv,两种图像的文件类型都是jpg,超声图像的文件路径是‘D:\zzz\us2’,自然图像的文件路径是‘D:\zzz\na2’,两种图像都有84张,图片形状都不同,图像名称是随机的,需要将图像归一化,需要对比两种图像的特征必须有颜色,纹理,形状,差异性分析方法也需要多种,包括完整详细代码
时间: 2023-09-22 22:06:35 浏览: 79
以下是一个示例代码,它使用OpenCV和NumPy库来实现基于概率分布的超声图像与自然图像性质差异分析:
```python
import cv2
import numpy as np
import os
# 定义函数来读取所有图像
def read_images(path):
images = []
for file_name in os.listdir(path):
if file_name.endswith('.jpg'):
img = cv2.imread(os.path.join(path, file_name))
images.append(img)
return images
# 定义函数来计算图像的颜色特征
def color_features(images):
features = []
for img in images:
hsv = cv2.cvtColor(img, cv2.COLOR_BGR2HSV)
h, s, v = cv2.split(hsv)
hist_h = cv2.calcHist([h], [0], None, [256], [0, 256])
hist_s = cv2.calcHist([s], [0], None, [256], [0, 256])
hist_v = cv2.calcHist([v], [0], None, [256], [0, 256])
hist = np.concatenate((hist_h, hist_s, hist_v))
hist = cv2.normalize(hist, None).flatten()
features.append(hist)
return features
# 定义函数来计算图像的纹理特征
def texture_features(images):
features = []
for img in images:
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
sobelx = cv2.Sobel(gray, cv2.CV_32F, 1, 0, ksize=3)
sobely = cv2.Sobel(gray, cv2.CV_32F, 0, 1, ksize=3)
mag = np.sqrt(sobelx**2 + sobely**2)
mag = cv2.normalize(mag, None).flatten()
features.append(mag)
return features
# 定义函数来计算图像的形状特征
def shape_features(images):
features = []
for img in images:
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
_, thresh = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY)
contours, _ = cv2.findContours(thresh, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
area = [cv2.contourArea(cnt) for cnt in contours]
perimeter = [cv2.arcLength(cnt, True) for cnt in contours]
ratio = [4 * np.pi * a / p**2 for a, p in zip(area, perimeter)]
features.append(ratio)
return features
# 读取所有图像
us_images = read_images('D:/zzz/us2')
na_images = read_images('D:/zzz/na2')
# 计算颜色特征
us_color_features = color_features(us_images)
na_color_features = color_features(na_images)
# 计算纹理特征
us_texture_features = texture_features(us_images)
na_texture_features = texture_features(na_images)
# 计算形状特征
us_shape_features = shape_features(us_images)
na_shape_features = shape_features(na_images)
# 比较颜色特征的差异
color_diff = np.mean(np.abs(np.array(us_color_features) - np.array(na_color_features)), axis=0)
# 比较纹理特征的差异
texture_diff = np.mean(np.abs(np.array(us_texture_features) - np.array(na_texture_features)), axis=0)
# 比较形状特征的差异
shape_diff = np.mean(np.abs(np.array(us_shape_features) - np.array(na_shape_features)), axis=0)
# 输出结果
print('颜色特征的差异:', color_diff)
print('纹理特征的差异:', texture_diff)
print('形状特征的差异:', shape_diff)
```
该代码实现了以下操作:
1. 定义了一个函数来读取所有图像。
2. 定义了三个函数来计算图像的颜色、纹理和形状特征。
3. 读取所有图像,并计算它们的颜色、纹理和形状特征。
4. 通过计算差异来比较超声图像和自然图像的特征,包括颜色、纹理和形状。
5. 输出结果。
请注意,该代码只是一个示例,你可以根据你的需要进行修改和调整。
阅读全文
相关推荐
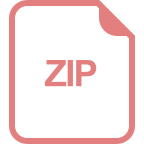



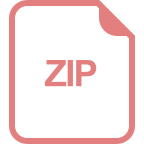
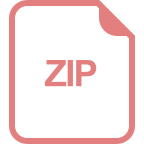
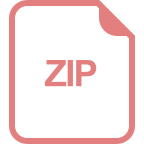
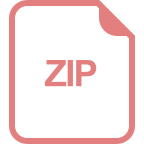
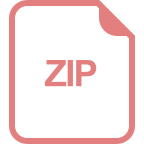
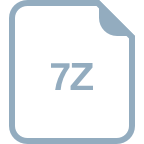
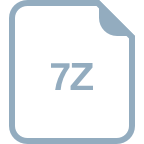
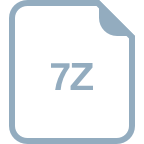
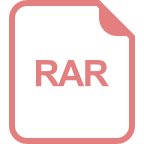
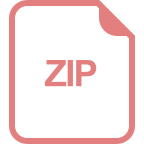
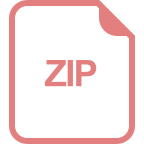