vue-quill-editor实现上传excel
时间: 2023-07-12 13:19:32 浏览: 59
`vue-quill-editor`是一个富文本编辑器组件,并不支持上传Excel文件,如果你需要实现上传Excel文件的功能,需要借助其他组件或库来实现。这里介绍一种使用`element-ui`组件实现上传Excel文件并将其内容显示在富文本编辑器中的方法。
首先,在模板中添加一个`el-upload`组件用于上传Excel文件,然后在`before-upload`方法中处理上传的文件,将其转换为JSON格式并保存在组件的`excelData`属性中。
```html
<template>
<div>
<el-upload
class="upload-excel"
action="#"
:before-upload="handleBeforeUpload"
:show-file-list="false"
>
<el-button size="small" type="primary">上传Excel</el-button>
</el-upload>
<quill-editor v-model="content"></quill-editor>
</div>
</template>
<script>
import { quillEditor } from 'vue-quill-editor';
export default {
components: {
quillEditor,
},
data() {
return {
content: '',
excelData: null,
};
},
methods: {
handleBeforeUpload(file) {
const reader = new FileReader();
reader.onload = e => {
const data = e.target.result;
const workbook = XLSX.read(data, { type: 'binary' });
const worksheet = workbook.Sheets[workbook.SheetNames[0]];
const json = XLSX.utils.sheet_to_json(worksheet);
this.excelData = json;
};
reader.readAsBinaryString(file);
},
},
};
</script>
```
上述代码中,使用了`XLSX`库来处理Excel文件。该库可以将Excel文件转换为JSON格式,方便我们在富文本编辑器中进行显示和编辑。
接下来,在`content`属性的`watch`方法中监听富文本编辑器内容的变化,如果内容中包含特定的关键字(例如“{{excel}}”),则将其替换为我们上传的Excel文件的内容。
```html
<template>
<div>
<el-upload
class="upload-excel"
action="#"
:before-upload="handleBeforeUpload"
:show-file-list="false"
>
<el-button size="small" type="primary">上传Excel</el-button>
</el-upload>
<quill-editor v-model="content"></quill-editor>
</div>
</template>
<script>
import { quillEditor } from 'vue-quill-editor';
export default {
components: {
quillEditor,
},
data() {
return {
content: '',
excelData: null,
};
},
watch: {
content(newVal) {
if (!newVal.includes('{{excel}}') || !this.excelData) return;
const table = this.formatTable(this.excelData);
const content = newVal.replace('{{excel}}', table);
this.content = content;
},
},
methods: {
handleBeforeUpload(file) {
const reader = new FileReader();
reader.onload = e => {
const data = e.target.result;
const workbook = XLSX.read(data, { type: 'binary' });
const worksheet = workbook.Sheets[workbook.SheetNames[0]];
const json = XLSX.utils.sheet_to_json(worksheet);
this.excelData = json;
};
reader.readAsBinaryString(file);
},
formatTable(data) {
const table = document.createElement('table');
const thead = document.createElement('thead');
const tbody = document.createElement('tbody');
const headers = Object.keys(data[0]);
const headerRow = document.createElement('tr');
headers.forEach(header => {
const th = document.createElement('th');
th.textContent = header;
headerRow.appendChild(th);
});
thead.appendChild(headerRow);
data.forEach(rowData => {
const tr = document.createElement('tr');
headers.forEach(header => {
const td = document.createElement('td');
td.textContent = rowData[header];
tr.appendChild(td);
});
tbody.appendChild(tr);
});
table.appendChild(thead);
table.appendChild(tbody);
return table.outerHTML;
},
},
};
</script>
```
在上述代码中,我们在`formatTable`方法中将JSON数据转换为HTML表格,然后在`watch`方法中将其替换到富文本编辑器的内容中。如果需要,你还可以进一步调整表格的样式以适应你的页面需求。
希望这些信息对你有所帮助。
相关推荐
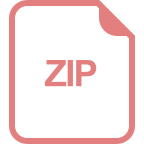
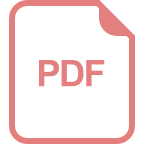














