如何将上述代码使用Django前后端分离
时间: 2024-04-26 22:23:48 浏览: 10
要将上述的问答系统使用Django实现前后端分离,可以采用Django REST framework来实现API接口,前端可以使用Vue.js等前端框架来调用API接口。
以下是一个简单的示例代码,使用Django REST framework实现上述问答系统:
1. 安装Django和Django REST framework
```bash
pip install django
pip install djangorestframework
```
2. 创建Django项目和应用
```bash
django-admin startproject qa_system
cd qa_system
python manage.py startapp api
```
3. 修改`qa_system/settings.py`文件,添加`rest_framework`和`api`应用
```python
INSTALLED_APPS = [
# ...
'rest_framework',
'api',
]
# ...
REST_FRAMEWORK = {
'DEFAULT_RENDERER_CLASSES': [
'rest_framework.renderers.JSONRenderer',
]
}
```
4. 在`api/models.py`文件中定义数据模型
```python
from django.db import models
class Question(models.Model):
question_text = models.CharField(max_length=200)
answer_text = models.CharField(max_length=200)
def __str__(self):
return self.question_text
```
5. 在`api/serializers.py`文件中定义序列化器
```python
from rest_framework import serializers
from .models import Question
class QuestionSerializer(serializers.ModelSerializer):
class Meta:
model = Question
fields = ['question_text', 'answer_text']
```
6. 在`api/views.py`文件中定义视图
```python
from rest_framework import generics
from .models import Question
from .serializers import QuestionSerializer
class QAView(generics.RetrieveAPIView):
queryset = Question.objects.all()
serializer_class = QuestionSerializer
lookup_field = 'question_text'
```
7. 在`qa_system/urls.py`文件中添加路由
```python
from django.urls import path, include
from api.views import QAView
urlpatterns = [
path('api/qa/<str:question_text>/', QAView.as_view(), name='qa'),
]
```
8. 运行Django服务器
```bash
python manage.py runserver
```
现在你可以在浏览器中访问`http://localhost:8000/api/qa/<question_text>/`来获取问答系统的答案,其中`<question_text>`为问题文本。你也可以在前端使用Vue.js等前端框架来调用该API接口,获取问答系统的答案。
相关推荐
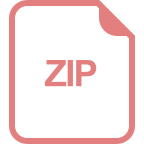
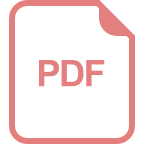














