JRadioButton.setIcon设置的按钮背景色不是透明的
时间: 2024-05-13 22:14:41 浏览: 107
JRadioButton.setIcon() 方法设置的是按钮的图标,而不是背景色。如果想要设置按钮的背景色为透明,可以使用 JRadioButton.setOpaque(false) 方法。这会将按钮的背景设置为透明,使得父容器的背景色或者上层组件的背景色可以透过按钮显示出来。例如:
```
JRadioButton radioButton = new JRadioButton("选项");
radioButton.setOpaque(false); // 设置按钮背景为透明
```
如果设置了按钮的背景图片,那么需要使用 setOpaque(false) 方法来确保背景图片可以正确地显示。
相关问题
1.设计一个包含三个单选按钮,背景色分别为红色、绿色和蓝色的GUI,效果如图所示。 要求: 1) 窗体标题命名为“颜色选择器”。 2) 使用边界布局管理器BorderLayout。 3) 使用单选按钮JRadioButton组件。 4) 添加事件监听器,实现背景色分别为红色、绿色和蓝色。
好的,我来为您解答这个问题。
首先,您需要引入Java Swing包,然后可以使用如下代码实现您的需求:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class ColorChooser extends JFrame implements ActionListener{
private JRadioButton redBtn;
private JRadioButton greenBtn;
private JRadioButton blueBtn;
private Container c;
public ColorChooser(){
super("颜色选择器");
c = getContentPane();
c.setLayout(new BorderLayout());
JPanel radioPanel = new JPanel();
radioPanel.setLayout(new GridLayout(1, 3));
redBtn = new JRadioButton("红色", true);
greenBtn = new JRadioButton("绿色");
blueBtn = new JRadioButton("蓝色");
ButtonGroup group = new ButtonGroup();
group.add(redBtn);
group.add(greenBtn);
group.add(blueBtn);
redBtn.addActionListener(this);
greenBtn.addActionListener(this);
blueBtn.addActionListener(this);
radioPanel.add(redBtn);
radioPanel.add(greenBtn);
radioPanel.add(blueBtn);
c.add(radioPanel, BorderLayout.NORTH);
setSize(300, 300);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setVisible(true);
}
@Override
public void actionPerformed(ActionEvent e) {
if (e.getSource() == redBtn) {
c.setBackground(Color.RED);
} else if (e.getSource() == greenBtn) {
c.setBackground(Color.GREEN);
} else if (e.getSource() == blueBtn) {
c.setBackground(Color.BLUE);
}
}
public static void main(String[] args){
new ColorChooser();
}
}
```
这段代码创建了一个继承自JFrame的窗口类ColorChooser,实现了ActionListener接口用于监听单选按钮的事件。在构造函数中,我们使用边界布局管理器BorderLayout将单选按钮放在窗口的北边,并将这三个单选按钮添加到同一个ButtonGroup中,以确保只有其中一个被选中。然后,我们使用了setSize()方法设置了窗口的大小,并使用setDefaultCloseOperation()方法设置了窗口的关闭操作。最后,我们调用setVisible()方法将窗口显示出来。
在actionPerformed()方法中,我们根据被选中的单选按钮设置窗口的背景色。
希望这个代码可以帮助到您!
java1.设计一个包含三个单选按钮,背景色分别为红色、绿色和蓝色的GUI,效果如图所示。 要求: 1) 窗体标题命名为“颜色选择器”。(4分) 2) 使用边界布局管理器BorderLayout。(4分) 3) 使用单选按钮JRadioButton组件。(7分) 4) 添加事件监听器,实现背景色分别为红色、绿色和蓝色。(15分)
以下是Java代码实现:
```
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.ButtonGroup;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JRadioButton;
public class ColorPicker extends JFrame implements ActionListener {
private JPanel colorPanel;
private JRadioButton redButton, greenButton, blueButton;
public ColorPicker() {
setTitle("颜色选择器");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(300, 300);
setLocationRelativeTo(null);
colorPanel = new JPanel();
add(colorPanel, BorderLayout.CENTER);
JPanel buttonPanel = new JPanel();
redButton = new JRadioButton("红色");
greenButton = new JRadioButton("绿色");
blueButton = new JRadioButton("蓝色");
ButtonGroup group = new ButtonGroup();
group.add(redButton);
group.add(greenButton);
group.add(blueButton);
redButton.addActionListener(this);
greenButton.addActionListener(this);
blueButton.addActionListener(this);
buttonPanel.add(redButton);
buttonPanel.add(greenButton);
buttonPanel.add(blueButton);
add(buttonPanel, BorderLayout.SOUTH);
}
@Override
public void actionPerformed(ActionEvent e) {
if (e.getSource() == redButton) {
colorPanel.setBackground(Color.RED);
} else if (e.getSource() == greenButton) {
colorPanel.setBackground(Color.GREEN);
} else if (e.getSource() == blueButton) {
colorPanel.setBackground(Color.BLUE);
}
}
public static void main(String[] args) {
ColorPicker picker = new ColorPicker();
picker.setVisible(true);
}
}
```
代码解释:
- 首先创建一个JFrame窗体,设置标题和关闭操作,大小为300x300,并居中显示。
- 创建一个JPanel作为颜色展示面板,添加到窗体的中央位置。
- 创建一个JPanel作为按钮面板,使用边界布局管理器BorderLayout,并添加到窗体的南部位置。
- 创建三个单选按钮JRadioButton,分别为“红色”、“绿色”、“蓝色”,并添加到按钮面板中。
- 使用ButtonGroup将按钮组合在一起,使它们只能选择其中一个。
- 为每个单选按钮添加事件监听器,当单选按钮被选中时,调用actionPerformed()方法,根据选中的按钮设置颜色展示面板的背景色。
- 在main()方法中创建ColorPicker对象,并将其设置为可见状态。
运行程序,即可看到一个颜色选择器界面,单选按钮可以选择不同的颜色,颜色展示面板的背景色会随着按钮的选择而改变。
阅读全文
相关推荐
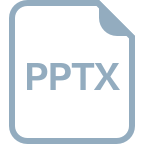
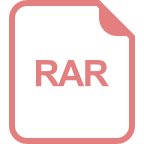
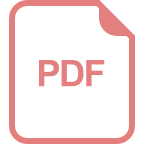







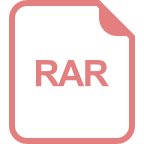
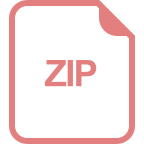
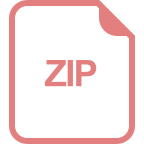