springboot freemarker 自定义列表循环标签 带网页标签的示例
时间: 2023-06-23 19:10:01 浏览: 116
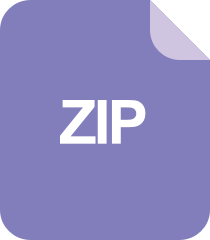
实现通过jfinal框架自动扫描freemarker的自定义标签.zip
下面是一个示例,展示如何在Spring Boot中使用FreeMarker自定义列表循环标签,并带有网页标签:
1. 创建一个自定义标签类CustomListTag,继承LoopTagSupport类,并实现doOnce方法和doEnd方法。
```java
import java.io.IOException;
import java.util.Map;
import javax.servlet.http.HttpServletRequest;
import org.springframework.web.servlet.support.RequestContextUtils;
import freemarker.core.Environment;
import freemarker.template.TemplateDirectiveBody;
import freemarker.template.TemplateDirectiveModel;
import freemarker.template.TemplateException;
import freemarker.template.TemplateModel;
import freemarker.template.TemplateModelException;
import freemarker.template.utility.DeepUnwrap;
public class CustomListTag extends LoopTagSupport implements TemplateDirectiveModel {
private int index = 0;
@Override
protected boolean process() throws TemplateModelException {
if (index >= size()) {
return false;
}
Environment env = getEnvironment();
TemplateDirectiveBody body = getBody();
if (body != null) {
try {
env.setVariable("index", getStart() + index);
env.setVariable("item", DeepUnwrap.unwrap(get(index)));
body.render(env.getOut());
} catch (IOException | TemplateException e) {
throw new RuntimeException(e);
}
}
index++;
return true;
}
@Override
public void doOnce(Map params, TemplateModel[] loopVars, TemplateDirectiveBody body) throws TemplateException, IOException {
super.doOnce(params, loopVars, body);
HttpServletRequest request = (HttpServletRequest) getEnvironment().getCustomAttribute("request");
String contextPath = request.getContextPath();
getEnvironment().setVariable("contextPath", getConfiguration().getObjectWrapper().wrap(contextPath));
}
@Override
public void doEnd(Environment env, Map params, TemplateModel[] loopVars, TemplateDirectiveBody body)
throws TemplateException, IOException {
env.setVariable("index", null);
env.setVariable("item", null);
env.setVariable("contextPath", null);
super.doEnd(env, params, loopVars, body);
}
}
```
2. 在Spring Boot配置类中注册自定义标签
```java
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.view.freemarker.FreeMarkerConfigurer;
@Configuration
public class FreeMarkerConfig {
@Bean
public FreeMarkerConfigurer freeMarkerConfigurer() {
FreeMarkerConfigurer configurer = new FreeMarkerConfigurer();
configurer.setTemplateLoaderPath("classpath:/templates/");
configurer.setDefaultEncoding("UTF-8");
Map<String, Object> variables = new HashMap<>();
variables.put("customList", new CustomListTag());
configurer.setFreemarkerVariables(variables);
return configurer;
}
}
```
3. 在FreeMarker模板中使用自定义标签
```html
<#list items as item>
<#if customList.first>
<ul>
</#if>
<li>${item}</li>
<#if customList.last>
</ul>
</#if>
</#list>
```
在这个示例中,我们将一个列表包装在自定义标签中,用<ul>和<li>标记包装它,并设置了一个变量contextPath,它包含了上下文路径,在模板中可以方便地使用。
阅读全文
相关推荐
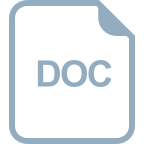
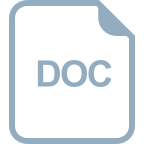

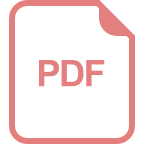
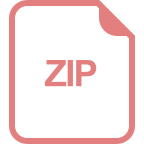
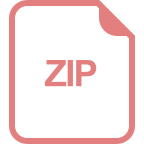
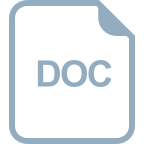
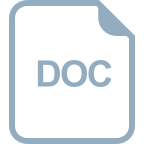
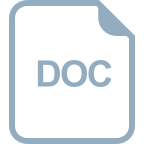
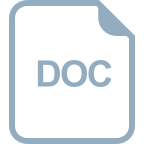
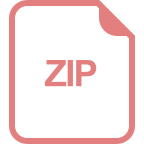
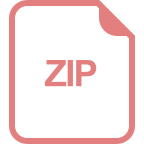
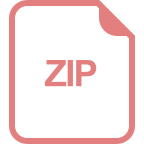
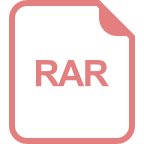
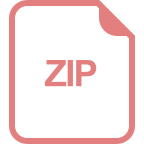