avformat_open_input失败 再去av_read_frame卡死
时间: 2023-10-01 13:05:01 浏览: 154
这个问题可能是由于音视频文件的格式不被FFmpeg所支持,或者文件损坏导致的。你可以检查一下文件格式是否正确,并且尝试使用其他音视频文件进行测试。另外,也可以尝试使用FFmpeg的命令行工具对该文件进行解码,看是否存在相同的问题。如果问题仍然存在,可以尝试使用调试工具对代码进行调试,查看具体的错误信息。
相关问题
avformat_open_input用法
avformat_open_input是FFmpeg中的一个函数,用于打开一个输入文件,并将其解析成一个AVFormatContext结构体,以便后续对文件进行读取操作。
函数原型如下:
```
int avformat_open_input(AVFormatContext **ps, const char *url, AVInputFormat *fmt, AVDictionary **options);
```
参数说明:
- ps:指向AVFormatContext结构体指针的指针,用于存储解析后的文件信息;
- url:输入文件的路径或URL;
- fmt:指定输入文件的格式,如果为NULL,则由FFmpeg自动检测文件格式;
- options:指向AVDictionary结构体指针的指针,用于设置解码器参数等选项。
函数返回值:
成功返回0,失败返回负数错误码。
使用示例:
```
#include <libavformat/avformat.h>
int main(int argc, char *argv[]) {
AVFormatContext *formatCtx = NULL;
int ret = avformat_open_input(&formatCtx, "input.mp4", NULL, NULL);
if (ret < 0) {
printf("Error: Could not open input file.\n");
return -1;
}
// 接下来可以对文件进行读取操作
avformat_close_input(&formatCtx);
return 0;
}
```
上述示例代码中,avformat_open_input函数打开了名为“input.mp4”的文件,并将解析后的文件信息存储在AVFormatContext结构体中。接下来可以使用AVFormatContext结构体中的函数进行读取操作,例如使用av_read_frame函数读取视频帧等。
最后需要使用avformat_close_input函数关闭文件并释放AVFormatContext结构体。
avformat_open_input函数详解
avformat_open_input 函数是 FFmpeg 中的一个重要函数,用于打开输入媒体文件,创建一个 AVFormatContext 结构体,并且为每个流分配一个 AVStream 结构体。该函数的使用方法如下:
1. 初始化 AVFormatContext 结构体
首先需要初始化一个 AVFormatContext 结构体,可以使用 avformat_alloc_context 函数来分配内存空间。
2. 打开输入文件
调用 avformat_open_input 函数来打开输入媒体文件,并将文件的信息存储在 AVFormatContext 结构体中。该函数的原型如下:
```c
int avformat_open_input(AVFormatContext **ps, const char *url, AVInputFormat *fmt, AVDictionary **options);
```
其中,参数 ps 是一个指向 AVFormatContext 指针的指针,url 是输入媒体文件的路径,fmt 是一个 AVInputFormat 结构体指针,用于指定输入媒体文件的格式,如果设置为 NULL,则会自动检测输入文件的格式。options 是一个 AVDictionary 结构体指针,用于设置一些额外的选项,例如设置输入缓冲区大小等。
3. 检查 AVFormatContext 结构体
调用 avformat_find_stream_info 函数来检查 AVFormatContext 结构体,并获取媒体文件的一些基本信息,例如流的数量、每个流的编码格式等等。该函数的原型如下:
```c
int avformat_find_stream_info(AVFormatContext *ic, AVDictionary **options);
```
其中,参数 ic 是一个指向 AVFormatContext 结构体的指针,options 是一个 AVDictionary 结构体指针,用于设置一些额外的选项。
4. 获取流的信息
遍历 AVFormatContext 结构体中的每个 AVStream 结构体,获取每个流的详细信息,例如编码格式、码率、时长等等。
下面是一个简单的示例代码,演示了如何使用 avformat_open_input 函数打开一个媒体文件,并获取每个流的信息:
```c
#include <libavformat/avformat.h>
int main(int argc, char* argv[]) {
AVFormatContext* formatContext = NULL;
int ret = avformat_open_input(&formatContext, "input.mp4", NULL, NULL);
if (ret < 0) {
printf("Failed to open input file!\n");
return -1;
}
ret = avformat_find_stream_info(formatContext, NULL);
if (ret < 0) {
printf("Failed to find stream info!\n");
return -1;
}
for (int i = 0; i < formatContext->nb_streams; i++) {
AVCodecParameters* codecParam = formatContext->streams[i]->codecpar;
printf("stream %d: codec_id=%d, codec_name=%s, bitrate=%lld, duration=%lld\n",
i, codecParam->codec_id, avcodec_get_name(codecParam->codec_id),
formatContext->bit_rate, formatContext->duration);
}
avformat_close_input(&formatContext);
return 0;
}
```
在这个示例代码中,我们使用 avformat_open_input 函数打开了一个名为 input.mp4 的媒体文件,然后遍历了 AVFormatContext 结构体中的每个流,并打印出每个流的编码格式、码率和时长等信息。
需要注意的是,avformat_open_input 函数只是打开了输入媒体文件,并创建了一个 AVFormatContext 结构体,但并没有开始解码媒体文件。要想解码媒体文件,需要使用其他的函数,例如 av_read_frame 函数来读取媒体文件的每一帧数据。
阅读全文
相关推荐
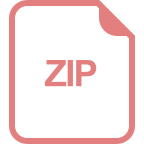
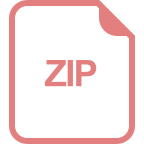
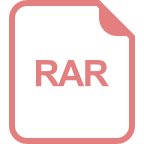













