用C语言写一段代码,统计一个字符串中的单词个数,字符串中可能出现,数字,所有格,标点符号
时间: 2023-10-27 10:07:02 浏览: 89
好的,可以使用以下 C 语言代码来实现:
```c
#include <stdio.h>
#include <ctype.h>
int count_words(char *s) {
int count = 0;
char *p = s;
while (*p != '\0') {
// 如果当前字符不为空格或标点符号,则继续向后遍历
if (!isspace(*p) && !ispunct(*p)) {
p++;
} else {
// 如果当前字符是空格或标点符号,并且前一个字符不是空格或标点符号,则单词个数加1
if (p > s && !isspace(*(p-1)) && !ispunct(*(p-1))) {
count++;
}
p++;
}
}
// 如果字符串末尾是字母,则单词个数加1
if (p > s && !isspace(*(p-1)) && !ispunct(*(p-1))) {
count++;
}
return count;
}
int main() {
char s[100];
printf("Please enter a string: ");
fgets(s, sizeof(s), stdin);
printf("The number of words in the string is: %d\n", count_words(s));
return 0;
}
```
其中,`isspace()` 和 `ispunct()` 函数用于判断字符是否为空格或标点符号,`p++` 语句用于向后遍历字符串,`if` 语句用于判断单词边界并统计单词个数。注意,`fgets()` 函数可以读取带空格的字符串,且在字符串末尾自动添加一个空字符 `\0`。
阅读全文
相关推荐
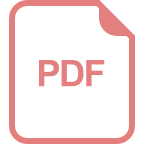
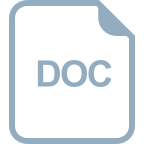
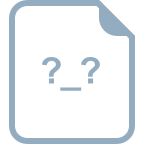















