请用C++完成这个实验:定义一个Cat类,有age、weight等成员变量,并有一个静态成员变量numOfCats,记录Cat的个体数目;静态成员函数getNumOfCats()用于读取numOfCats。在main函数中定义几个Cat类的对象,并调用getNumOfCats()函数得到Cat对象的个数,显示出这个个数。体会静态数据成员和静态成员函数的用法。
时间: 2023-05-26 14:01:35 浏览: 128
#include <iostream>
using namespace std;
class Cat {
public:
Cat(int age, int weight) {
this->age = age;
this->weight = weight;
numOfCats++;
}
static int getNumOfCats() {
return numOfCats;
}
private:
int age;
int weight;
static int numOfCats;
};
int Cat::numOfCats = 0;
int main() {
Cat cat1(2, 3);
Cat cat2(3, 4);
Cat cat3(4, 5);
cout << "Cat对象的个数为:" << Cat::getNumOfCats() << endl;
return 0;
}
相关问题
用C++编写:定义一个Cat类,有成员age、weight等属性,以及成员函数grow(int years)对age加上years和showInfo函数显示对象属性,实现并测试这个类。
好的,以下是Cat类的定义和实现的C语言代码:
```c
#include <stdio.h>
typedef struct {
int age;
int weight;
} Cat;
void grow(Cat* cat, int years) {
cat->age += years;
}
void showInfo(Cat* cat) {
printf("Age: %d, Weight: %d\n", cat->age, cat->weight);
}
int main() {
Cat myCat = {2, 5};
showInfo(&myCat); // 输出Age: 2, Weight: 5
grow(&myCat, 3);
showInfo(&myCat); // 输出Age: 5, Weight: 5
return 0;
}
```
在上述代码中,我们使用了C语言中的结构体来定义Cat类,并且定义了grow和showInfo函数,分别实现对age属性的加法操作和输出属性信息的功能。
在main函数中,我们首先创建了一个age为2,weight为5的Cat对象myCat,并使用showInfo函数输出它的属性信息。接着,我们使用grow函数将myCat的age属性加上了3,再一次使用showInfo函数输出属性信息,可以看到age属性已经变为了5。
以上就是Cat类的C语言实现和测试。
C++创建一个静态成员变量并在外部赋值
在C++中,可以通过在类中声明静态成员变量,并在类外部进行定义和赋值来创建静态成员变量。例如,假设有一个类Foo,需要创建一个名为s_count的静态成员变量并在外部进行赋值,可以按照以下步骤操作:
1. 在类定义中声明静态成员变量:
```c++
class Foo {
public:
static int s_count; //声明静态成员变量s_count
};
```
2. 在类外部进行定义:
```c++
int Foo::s_count; //定义静态成员变量s_count
```
3. 在类外部进行赋值:
```c++
Foo::s_count = 10; //赋值静态成员变量s_count的值为10
```
注意,静态成员变量可以被所有该类的对象共享,因此在任何一个对象中修改它的值都会影响到其他对象。
相关推荐
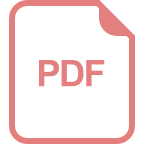
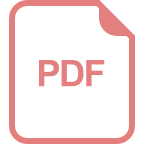
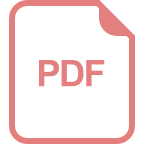












