帮我用ARM写接受键盘发出的按键,并把对应按键的数值显示在pc机的串口软件上用于STM32F103,写简单些
时间: 2024-05-10 13:15:54 浏览: 71
以下是一个简单的代码示例,可以接收键盘按键并将其对应的ASCII码值发送到串口。请注意,此代码仅处理单个按键的按下和释放。
```c
#include "stm32f10x.h"
int main(void)
{
// 初始化串口
USART_InitTypeDef USART_InitStructure;
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1 | RCC_APB2Periph_GPIOA, ENABLE);
// 配置USART1 Tx (PA9) 为复用推挽输出模式
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// 配置USART1 Rx (PA10) 为浮空输入模式
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// 配置串口参数
USART_InitStructure.USART_BaudRate = 115200;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
// 使能串口
USART_Cmd(USART1, ENABLE);
// 初始化GPIO
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// 循环检测键盘按键
while (1)
{
if (GPIO_ReadInputDataBit(GPIOA, GPIO_Pin_0) == RESET)
{
// 发送按键对应的ASCII码值
USART_SendData(USART1, 'A');
while (USART_GetFlagStatus(USART1, USART_FLAG_TXE) == RESET);
USART_ClearFlag(USART1, USART_FLAG_TXE);
// 等待按键释放
while (GPIO_ReadInputDataBit(GPIOA, GPIO_Pin_0) == RESET);
}
}
}
```
在此示例中,我们使用USART1来与PC机的串口通信。我们将PA9配置为USART1的Tx引脚,将PA10配置为USART1的Rx引脚。我们还将PA0配置为输入上拉,以检测键盘按键的状态。
在主循环中,我们不断检测PA0引脚的状态。如果它被按下,我们将字母“A”的ASCII码值发送到串口。然后等待按键释放。
阅读全文
相关推荐
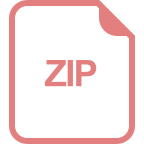
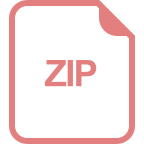
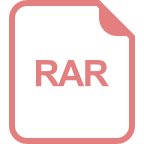
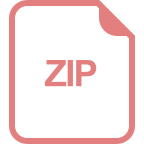
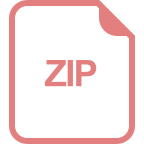
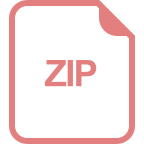
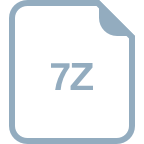
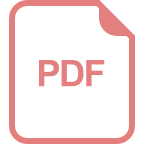
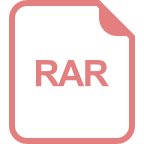
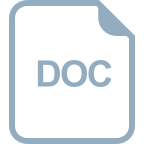
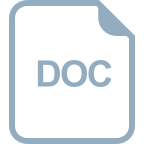
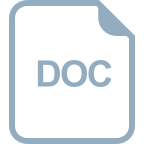
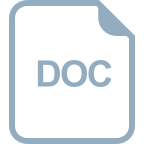
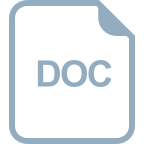
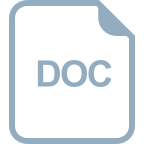
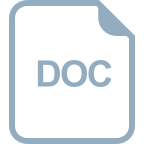