1.给出进程调度的算法描述(如基于动态优先级和时间片轮转调度算法的 描述)。 2.用C语言设计一个对n个并发进程进行调度的程序,每个进程由一个进程控制块(PCB)结构表示,该进程控制块应包括下述信息:进程标识ID、进程优先数PRIORITY(并规定优先数与优先权成正比)、时间片数CHIP、进程已经占 用CPU的时间CPUTIME,进程还需要运行的时间ALLTIME(当进程运行完毕 时,其值为0)、进程的状态STATE(为简化起见。设每个进程处于运行E(excec uting)、就绪R(ready)和完成F(finish)三种状态之一,并假设起始状态都是就绪状态R。),以及进程队列指针NEXT(用来将PCB排成队列)等,可按照调度 算法的不同而增删。 3.调度程序应当包含2种不同的调度算法,运行时可以任选一种,以利于各种方法的分析和比较。 4.程序应能显示或打印各种进程状态和参数变化情况,便于观察。即要显示每个时间片内各进程的情况,并且指出运行进程及就绪和阻塞队列中的内容。
时间: 2023-12-03 18:43:33 浏览: 98
1. 基于动态优先级的算法描述:
每个进程有一个动态优先级,当一个进程等待一段时间后,它的优先级会降低。当进程被分配到CPU时,它的优先级将被提高。每次选择最高优先级的进程来运行。
时间片轮转调度算法描述:
每个进程被分配一个时间片,当进程用完时间片后,会被放回就绪队列,并且下一个进程会被分配一个时间片来运行。
2. C语言程序设计:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_PROCESS 10
typedef enum { E, R, F } State;
typedef struct ProcessControlBlock {
int ID;
int PRIORITY;
int CHIP;
int CPUTIME;
int ALLTIME;
State STATE;
struct ProcessControlBlock* NEXT;
} PCB;
// 初始化进程控制块
void initPCB(PCB* pcb, int id, int priority, int chip, int alltime) {
pcb->ID = id;
pcb->PRIORITY = priority;
pcb->CHIP = chip;
pcb->CPUTIME = 0;
pcb->ALLTIME = alltime;
pcb->STATE = R;
pcb->NEXT = NULL;
}
// 将进程插入队列
void insertPCB(PCB** queue, PCB* pcb) {
if (*queue == NULL) {
*queue = pcb;
} else {
PCB* p = *queue;
while (p->NEXT != NULL) {
p = p->NEXT;
}
p->NEXT = pcb;
}
}
// 从队列中删除进程
PCB* removePCB(PCB** queue, int id) {
PCB* p = *queue;
PCB* pre = NULL;
while (p != NULL) {
if (p->ID == id) {
if (pre == NULL) {
*queue = p->NEXT;
} else {
pre->NEXT = p->NEXT;
}
return p;
}
pre = p;
p = p->NEXT;
}
return NULL;
}
// 选择最高优先级的进程
PCB* selectHighestPriority(PCB** queue) {
PCB* p = *queue;
PCB* maxP = p;
while (p != NULL) {
if (p->PRIORITY > maxP->PRIORITY) {
maxP = p;
}
p = p->NEXT;
}
removePCB(queue, maxP->ID);
return maxP;
}
// 时间片轮转调度算法
PCB* timeSlice(PCB** queue) {
PCB* p = *queue;
*queue = p->NEXT;
p->NEXT = NULL;
return p;
}
// 打印进程信息
void printPCB(PCB* pcb) {
printf("ID: %d, PRIORITY: %d, CHIP: %d, CPUTIME: %d, ALLTIME: %d, STATE: %d\n", pcb->ID, pcb->PRIORITY, pcb->CHIP, pcb->CPUTIME, pcb->ALLTIME, pcb->STATE);
}
// 打印队列信息
void printQueue(PCB* queue) {
if (queue == NULL) {
printf("Queue is empty!\n");
} else {
PCB* p = queue;
while (p != NULL) {
printPCB(p);
p = p->NEXT;
}
}
}
int main() {
int n;
printf("Enter the number of processes: ");
scanf("%d", &n);
PCB processes[MAX_PROCESS];
PCB* readyQueue = NULL;
PCB* finishQueue = NULL;
// 初始化进程
for (int i = 0; i < n; i++) {
int priority, chip, alltime;
printf("Enter the priority, chip and alltime of process %d: ", i + 1);
scanf("%d %d %d", &priority, &chip, &alltime);
initPCB(&processes[i], i + 1, priority, chip, alltime);
insertPCB(&readyQueue, &processes[i]);
}
int time = 0;
int algorithm;
printf("Enter the scheduling algorithm (1 for dynamic priority, 2 for time slice): ");
scanf("%d", &algorithm);
while (finishQueue != NULL && finishQueue->ALLTIME > 0) {
if (algorithm == 1) {
PCB* p = selectHighestPriority(&readyQueue);
p->STATE = E;
p->CPUTIME++;
p->ALLTIME--;
if (p->ALLTIME == 0) {
p->STATE = F;
insertPCB(&finishQueue, p);
} else {
if (p->CHIP > 0) {
p->CHIP--;
insertPCB(&readyQueue, p);
} else {
p->PRIORITY--;
insertPCB(&readyQueue, p);
}
}
} else if (algorithm == 2) {
if (readyQueue == NULL) {
printf("Time: %d, Queue is empty!\n", time);
} else {
PCB* p = timeSlice(&readyQueue);
p->STATE = E;
p->CPUTIME++;
p->ALLTIME--;
if (p->ALLTIME == 0) {
p->STATE = F;
insertPCB(&finishQueue, p);
} else {
insertPCB(&readyQueue, p);
}
}
}
printf("Time: %d\n", time);
printf("Running process: ");
if (finishQueue == NULL) {
printf("None\n");
} else {
printPCB(finishQueue);
}
printf("Ready queue: ");
printQueue(readyQueue);
printf("Finish queue: ");
printQueue(finishQueue);
time++;
}
return 0;
}
```
3. 两种不同的调度算法已经在上面的程序中实现了。
4. 程序会在每个时间片内打印进程的状态和队列的内容,方便观察。
相关推荐
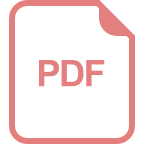
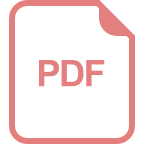















